HashMap in Java vs. TreeMap in Java: What's the Difference?

Edited by Aimie Carlson || By Janet White || Published on February 16, 2024
HashMap in Java is a hash table-based, unordered collection, while TreeMap is a Red-Black tree-based, sorted collection.
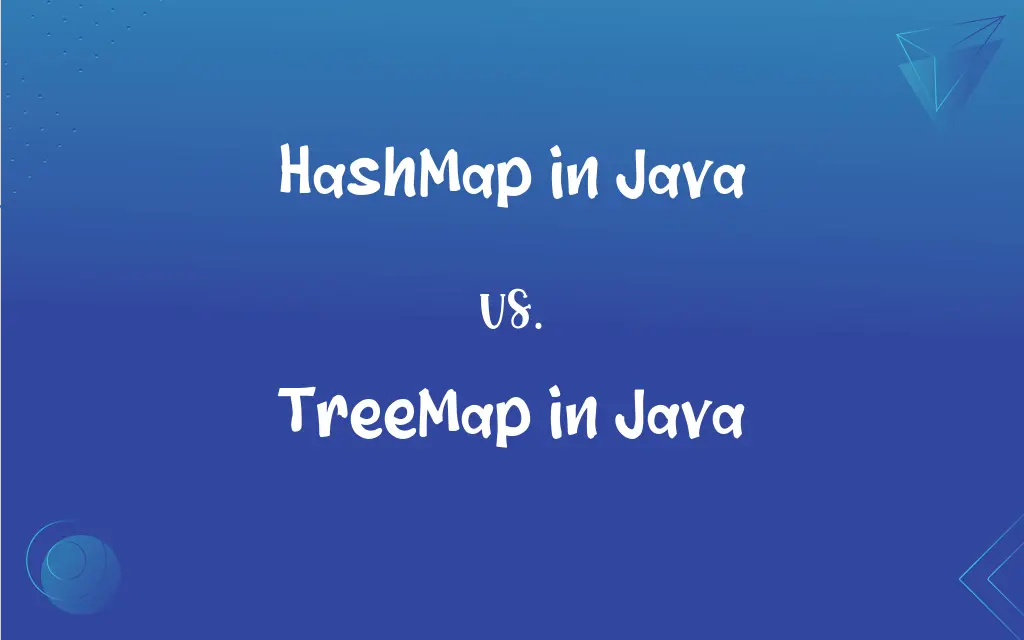
Key Differences
HashMap in Java is based on the hash table structure, allowing it to offer constant-time performance for basic operations like add, remove, and find, provided the hash function is well-designed. Conversely, TreeMap in Java is based on a Red-Black tree structure, which provides guaranteed log(n) time cost for the same operations, but with the additional feature of maintaining an order.
A key characteristic of a HashMap is that it does not maintain any order of its elements. This means that when iterating over a HashMap, the order of elements can be unpredictable. On the other hand, a TreeMap automatically sorts its elements based on their natural ordering or a custom Comparator, making it suitable for applications where sorted data is needed.
When it comes to null values, HashMap allows for one null key and multiple null values, making it more flexible in handling cases where data might be incomplete. In contrast, TreeMap does not allow null keys (as it cannot be sorted), but it can have null values, which suits scenarios where sorting of keys is crucial.
The internal working of a HashMap involves using the hashCode() method of objects, which can lead to a situation called 'hash collision' where two keys have the same hash code. TreeMap doesn’t use hashCode() but relies on compareTo() (or compare() from Comparator), thus avoiding hash collisions but requiring objects to be comparable.
In terms of performance, while both HashMap and TreeMap offer efficient data storage and retrieval, the choice between them depends on specific requirements: HashMap for speed in non-sorted operations, and TreeMap for sorted data operations.
ADVERTISEMENT
Comparison Chart
Underlying Structure
Hash table
Red-Black tree
Order of Elements
Unordered
Sorted according to natural ordering or Comparator
Null Key Support
Allows one null key
Does not allow null keys
Performance
Constant-time performance for basic operations
Log(n) time performance for basic operations
Handling of Collisions
Possible hash collisions
No hash collisions; relies on compareTo/compare
ADVERTISEMENT
HashMap in Java and TreeMap in Java Definitions
HashMap in Java
HashMap offers constant-time performance for get() and put() operations.
The HashMap provided fast retrieval of employee details.
TreeMap in Java
Provides log(n) time performance for most operations like add, remove, and containsKey.
Searching in our TreeMap of orders was efficient, thanks to its log(n) time complexity.
HashMap in Java
Allows one null key and multiple null values.
In our HashMap, we stored a null key with some default settings.
TreeMap in Java
TreeMap is based on Red-Black tree structure.
The TreeMap used a Red-Black tree, ensuring balanced data for quick access.
HashMap in Java
A collection that stores key-value pairs in a hash table.
We used a HashMap to store each student's ID and name.
TreeMap in Java
Suitable for applications where sorted data is needed.
We used TreeMap for our leaderboard to keep scores sorted in descending order.
HashMap in Java
Prone to hash collisions.
We had to handle hash collisions when multiple usernames had the same hash code in our HashMap.
TreeMap in Java
Does not allow null keys but allows multiple null values.
We could not use null as a key in our TreeMap of product prices.
HashMap in Java
Does not maintain any order of its elements.
Iterating over the HashMap yielded the elements in no particular order.
TreeMap in Java
A map implementation that keeps its entries sorted according to natural ordering or a custom Comparator.
Our TreeMap automatically sorted the customer names in alphabetical order.
FAQs
How does HashMap handle null values?
HashMap allows one null key and multiple null values.
Can TreeMap have null keys?
No, TreeMap cannot have null keys because keys need to be comparable for sorting.
What is a TreeMap in Java?
A TreeMap is a Red-Black tree-based implementation of the Map interface, which keeps its elements sorted.
What is a HashMap in Java?
A HashMap is a hash table-based implementation of the Map interface, providing fast access to elements.
What is the time complexity of TreeMap operations?
TreeMap provides log(n) time performance for basic operations like add, remove, and containsKey.
Can TreeMap handle hash collisions?
TreeMap does not face hash collisions as it is based on a tree structure and uses compareTo/compare for ordering.
How does HashMap deal with hash collisions?
HashMap handles hash collisions by maintaining a list of entries for each hash.
Why would you use a TreeMap?
Use a TreeMap when you need sorted access to data, such as in alphabetical ordering of keys.
Can a HashMap be sorted?
A HashMap itself cannot be sorted; you would need to use another collection like TreeMap for sorting.
What is the performance of HashMap in terms of time complexity?
HashMap offers constant-time performance for basic operations like get and put.
Which is faster for non-sorted operations, HashMap or TreeMap?
HashMap is generally faster for non-sorted operations due to its hash table implementation.
Is it possible to customize the sorting in TreeMap?
Yes, you can customize sorting in TreeMap by providing a custom Comparator.
Can you store an unordered collection in TreeMap?
No, TreeMap inherently orders its elements; for an unordered collection, use HashMap.
Is TreeMap sorted?
Yes, TreeMap maintains its elements in sorted order, either by natural ordering or a custom Comparator.
Is HashMap ordered?
No, HashMap does not maintain any order for its elements.
Are elements in a TreeMap automatically sorted?
Yes, elements in a TreeMap are automatically sorted.
What happens in a HashMap when two keys have the same hashCode?
In a HashMap, if two keys have the same hashCode, they are stored in a linked list under the same bucket.
What type of keys can be used in a TreeMap?
Keys in a TreeMap must be comparable, either naturally (like String, Integer) or through a Comparator.
Which is more suitable for applications requiring frequent insertions and deletions?
HashMap is generally more suitable for frequent insertions and deletions due to its constant-time performance.
How does TreeMap compare to HashMap in terms of memory usage?
TreeMap typically uses more memory than HashMap due to its tree structure.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
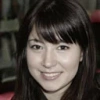