ref in C# vs. out in C#: What's the Difference?

Edited by Aimie Carlson || By Janet White || Published on February 23, 2024
ref in C# passes an argument by reference, allowing it to be both read and modified, while out is for passing arguments that are only meant to be initialized or written to.
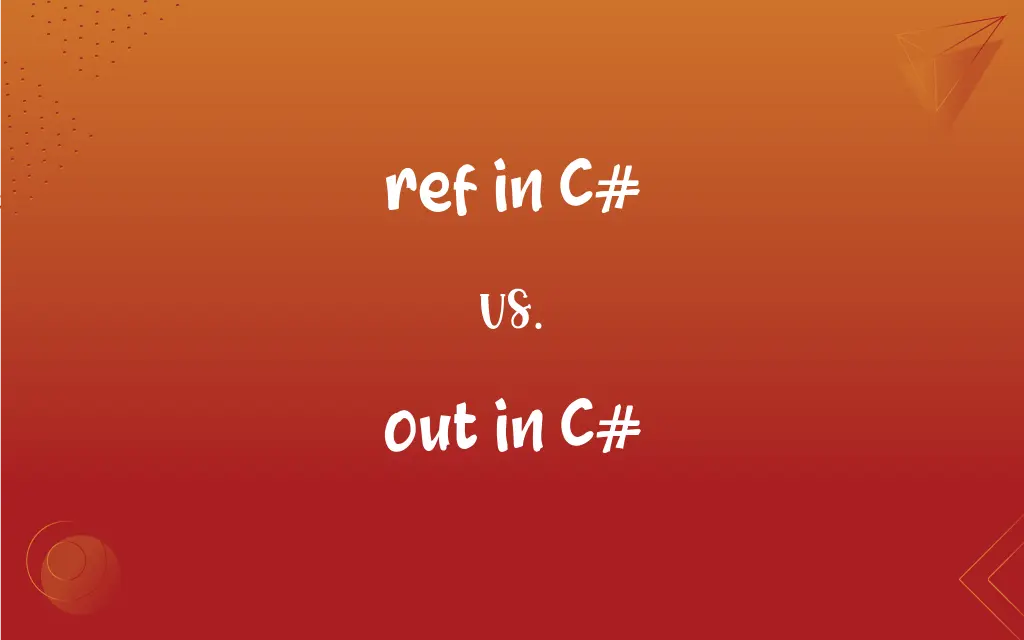
Key Differences
ref in C# is used to pass arguments to methods by reference, not by value, meaning any changes to the parameter inside the method affect the original argument. out is also for passing arguments by reference, but it's used when the intention is solely to return a value from a method, not to read the argument's initial value.
When using ref, the variable passed must be initialized before it's passed to the method. This is because the method receiving a ref parameter can read and modify the value. In contrast, variables passed with out do not need to be initialized, as the method is expected to assign them before returning.
ref parameters are versatile in that they allow a method to both read and write the value of the argument. This can be useful in scenarios where the method needs to modify the existing state of an argument. On the other hand, out parameters are designed for scenarios where a method needs to output more than one value, but doesn't need any initial input from the arguments.
A method with a ref parameter must recognize that the argument could have a value, and therefore must account for this in its logic. However, a method with an out parameter can assume it's responsible for setting the argument's value, simplifying some method logic.
Using ref and out both imply that the method will have side effects on the passed argument. However, the ref keyword indicates a two-way communication (read and write) between the method and the caller, whereas out signifies a one-way communication (write only) from the method to the caller.
ADVERTISEMENT
Comparison Chart
Initialization Required
Must be initialized before use
Does not require initialization
Method Usage
Can read and modify the value
Only sets the value
Typical Use Case
When a method modifies an existing value
When a method outputs a new value
Parameter Communication
Two-way (read/write)
One-way (write only)
Safety
Requires careful handling to avoid unintended modifications
Safer as it avoids using uninitialized data
ADVERTISEMENT
ref in C# and out in C# Definitions
ref in C#
With ref, both the method and caller can read and modify the argument.
Void Swap(ref int a, ref int b) { int temp = a; a = b; b = temp; }
out in C#
'out' in C# designates parameters that are intended to return values from methods.
Void GetCoordinates(out int x, out int y) { x = 0; y = 0; }
ref in C#
'ref' in C# is used for two-way communication between a method and its caller.
Void Calculate(ref int x) { x *= x; }
out in C#
With out, the method must assign a value before it returns.
Void GetStats(out int max, out int min) { max = 100; min = 1; }
ref in C#
'ref' requires the argument to be initialized to ensure predictable method behavior.
Int value = 5; ModifyValue(ref value);
out in C#
'out' is used for outputting values from methods without needing prior initialization.
Bool TryParse(string s, out int result) { return int.TryParse(s, out result); }
ref in C#
'ref 'indicates that the argument is not just passed but also potentially modified.
Void Increment(ref int count) { count++; }
out in C#
Parameters with out are exclusively for receiving values from the method.
Void Divide(int a, int b, out int quotient) { quotient = a / b; }
ref in C#
'ref' passes a variable by reference, allowing changes in the method to affect the original.
Void UpdateNumber(ref int num) { num = 10; }
out in C#
'out' parameters simplify methods that primarily return values.
Void TryGetValue(string key, out string value) { value = example; }
FAQs
What is ref in C#?
ref is a keyword for passing arguments by reference, allowing modifications.
When should ref be used in C#?
Use ref when a method needs to modify an existing variable's value.
What is out in C#?
out is a keyword for passing arguments by reference, primarily for outputting values.
Can ref parameters be null in C#?
Yes, if the reference type variable is initialized to null.
Are out parameters automatically initialized in methods?
The method must assign them before it returns.
Must ref parameters be initialized?
Yes, variables passed with ref must be initialized first.
Is ref or out more efficient in C#?
Efficiency depends on the use case; out can be more efficient for output-only scenarios.
What types of variables can be passed with ref?
Any variable type, including value and reference types.
Do out parameters require initialization?
No, out parameters do not need to be initialized before passing.
Can a method have both ref and out parameters in C#?
Yes, a method can have a mix of ref and out parameters.
How does ref affect method readability?
ref can make understanding a method's effect on parameters clearer.
What's the main use of out in C#?
out is used when a method needs to return additional values.
How does ref handle memory in C#?
ref passes a reference to the memory location, not the actual value.
Is ref or out more common in C#?
Both are common, but out is particularly popular in Try-pattern methods.
What error can occur with incorrect ref usage?
Incorrect ref usage can lead to unexpected modifications to variables.
How do ref and out improve code functionality?
They provide more flexibility in returning and modifying data in methods.
Is out used in Try-pattern methods in C#?
Yes, out is commonly used in Try-pattern methods like int.TryParse.
Can out parameters be used with reference types?
Yes, out can be used with both value and reference types.
Does using out imply multiple return values?
Yes, it's often used to return multiple values from a method.
Can out parameters lead to runtime errors?
If out parameters are not assigned in the method, it causes a compile-time error.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
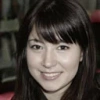