extends keywords in Java vs. implements keywords in Java: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 26, 2024
The 'extends' is used for inheriting from a class, while 'implements' is for applying an interface in Java.
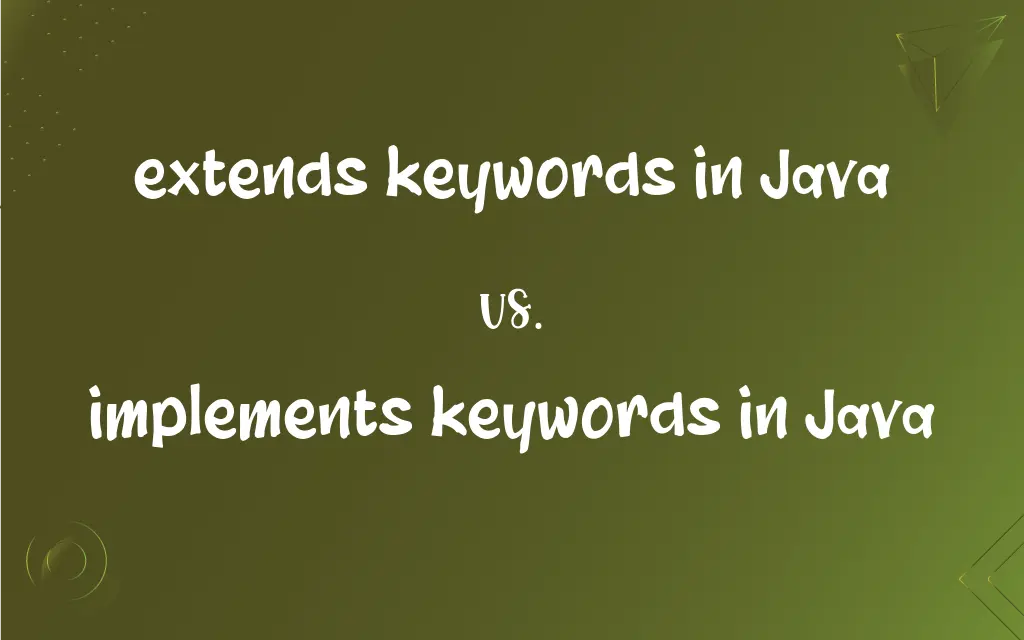
Key Differences
The 'extends' keyword in Java is used for class inheritance, meaning one class inherits the properties and methods of another. 'implements', however, is used when a class wants to implement an interface, agreeing to perform specific functions declared by the interface.
When using 'extends', a class extends another class, acquiring its attributes and behaviors. In contrast, 'implements' allows a class to implement an interface, which is a completely abstract class that defines a set of methods that the implementing class must have.
Java supports single inheritance using 'extends', meaning a class can only extend one other class. However, with 'implements', a class can implement multiple interfaces, providing greater flexibility in class design.
The 'extends' is often used to provide a concrete implementation of an abstract class. On the other hand, 'implements' is used to provide concrete implementations of all the abstract methods defined in an interface.
The use of 'extends' creates a strict hierarchy, as the child class inherits from one parent class. In contrast, 'implements' allows for a more flexible architecture, as a class can adhere to multiple contracts or behaviors as defined by interfaces.
ADVERTISEMENT
Comparison Chart
Usage
For class inheritance
For implementing interfaces
Inheritance Type
Single (one parent class)
Multiple (multiple interfaces)
Required Implementation
Inherits concrete and abstract methods
Must implement all abstract methods
Flexibility
Less flexible (single inheritance)
More flexible (multiple interfaces)
Design Purpose
Extending class functionality
Adhering to specific behaviors/interfaces
ADVERTISEMENT
extends keywords in Java and implements keywords in Java Definitions
extends keywords in Java
Enables polymorphic behavior in subclasses.
Class Bird extends Animal { void sing() { /* ... */ } }
implements keywords in Java
Defines specific behaviors from interfaces.
Class Timer implements Runnable { public void run() { /* ... */ } }
extends keywords in Java
Inherits constructors from the parent class.
Class Manager extends Employee { Manager() { super(); } }
implements keywords in Java
Enforces method definitions from an interface.
Class Car implements Vehicle { void drive() { /* ... */ } }
extends keywords in Java
Inherits fields from the parent class.
Class Triangle extends Shape { double area = base * height / 2; }
implements keywords in Java
Offers design flexibility with multiple behaviors.
Class NewsReader implements Reader, Sharable { /* ... */ }
extends keywords in Java
Allows overriding methods of the superclass.
Class ElectricCar extends Car { void drive() { /* ... */ } }
implements keywords in Java
Allows implementing multiple interfaces.
Class SmartPhone implements Camera, GPS { /* ... */ }
extends keywords in Java
Establishes a subclass relationship.
Class Dog extends Animal {}
implements keywords in Java
Requires implementation of all abstract methods.
Class MyList implements List { void add(Object obj) { /* ... */ } }
FAQs
Can a class extend multiple classes in Java?
No, Java supports single inheritance, so a class can extend only one class.
What does 'extends' do in Java?
'extends' is used for inheriting from a superclass.
Does 'extends' support method overriding?
Yes, methods of the superclass can be overridden in the subclass.
Can 'extends' and 'implements' be used together?
Yes, a class can extend another class and implement interfaces simultaneously.
What is the purpose of 'implements' in Java?
'implements' is used to apply an interface's methods in a class.
Can a class implement multiple interfaces?
Yes, a class can implement multiple interfaces in Java.
How does 'implements' affect class design?
It allows for more flexible design by incorporating multiple behaviors from different interfaces.
What happens if a class does not implement all methods of an interface?
The class must be declared abstract, or it will result in a compilation error.
Are private methods of the superclass inherited?
No, private methods are not inherited in subclasses.
Can 'extends' be used with interfaces?
Yes, an interface can extend another interface but cannot extend a class.
Can a class implement an interface without methods?
Yes, but it is not common practice.
What is inherited when a class extends another class?
The subclass inherits all public and protected members of the superclass.
Are constructors inherited through 'extends'?
Constructors are not inherited but can be accessed via 'super()' if they are public or protected.
Can interfaces extend other interfaces?
Yes, interfaces can extend other interfaces in Java.
Is it mandatory for a class to implement all interface methods?
Yes, unless the class is declared abstract.
How do 'extends' and 'implements' contribute to Java’s OOP features?
They enable inheritance and polymorphism, key features of OOP.
Can an abstract class use 'extends'?
Yes, an abstract class can extend another class or abstract class.
What are the limitations of using 'extends'?
The main limitation is single inheritance; a class cannot extend more than one class.
Do 'implements' and 'extends' support polymorphism?
Yes, both support polymorphism in different ways.
Is it possible to override interface methods?
Yes, interface methods can be overridden in the implementing class.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
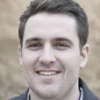
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
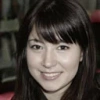