Delegates in C# vs. Events in C#: What's the Difference?

Edited by Harlon Moss || By Janet White || Published on February 7, 2024
Delegates in C# are object-oriented, type-safe function pointers, allowing methods to be passed as parameters; events in C# are special delegates designed for implementing the Observer pattern, encapsulating event handling.
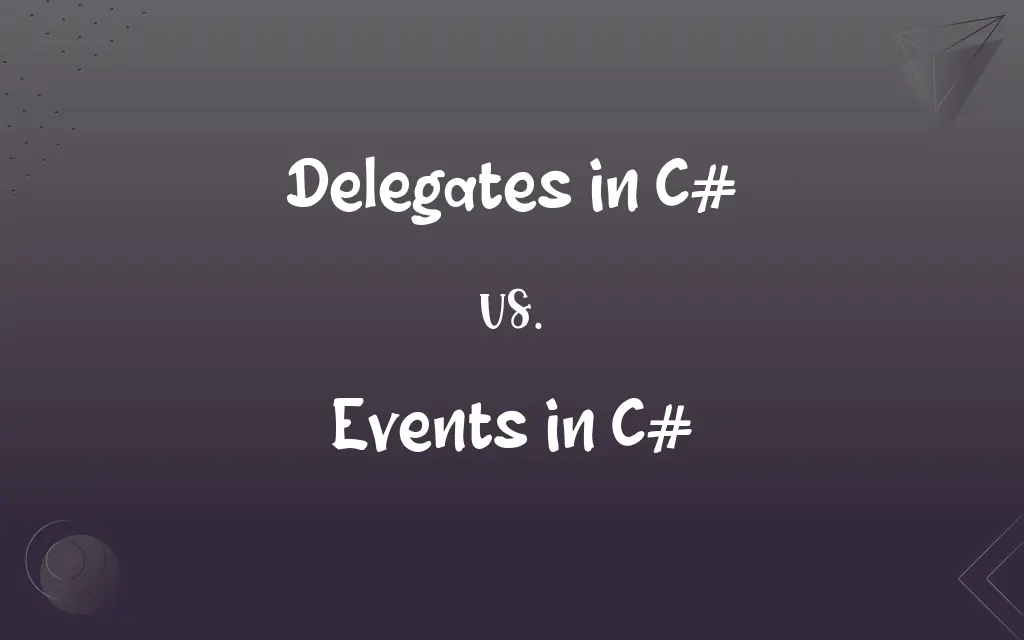
Key Differences
Delegates in C# are akin to function pointers in C and C++, but they are fully object-oriented and type-safe. They allow methods to be stored as variables and passed as arguments. In contrast, events in C# are a form of delegates specifically tailored for implementing event-driven programming. An event is a mechanism by which a class can notify its clients of something interesting that has occurred.
Delegates in C# provide a way to define callback methods. They can hold references to one or more methods and can be invoked dynamically at runtime. This provides flexibility in code design. On the other hand, events in C# are essentially a wrapper around a delegate, providing a more controlled way of accessing the delegate. Events are typically public members of a class and are used to signal subscribers when something of interest happens in or to that object.
In the context of delegates, they can be multicast, meaning a single delegate instance can reference multiple methods. This is useful for scenarios where you want to notify multiple methods about a certain task being completed. Events, conversely, are based on delegates but are used in a publish-subscribe model. They allow a class to expose notifications while maintaining encapsulation, preventing external code from directly invoking the delegate.
Delegates in C# are more generic and can be used for various purposes, not limited to event handling. They offer a flexible way to pass methods as arguments, allowing dynamic invocation. Events, in comparison, are a specialized use case of delegates. They are specifically designed to facilitate a communication contract between objects in a way that supports loose coupling, making them essential for event-driven programming.
Delegates in C# can be thought of as the foundation upon which events are built. While delegates offer the functionality to hold and invoke methods, events add an additional layer of abstraction and control. Events are a syntactic sugar over delegates, providing a way to implement the Observer pattern in a language-supported way, making them more intuitive and safe for event handling scenarios.
ADVERTISEMENT
Comparison Chart
Definition
Type-safe function pointers, hold method references.
Specialized delegates for event handling.
Usage
General-purpose, used for callbacks, and dynamic invocation.
Specific to event-driven programming, managing notifications.
Flexibility
Can reference multiple methods (multicast).
Typically reference a single invocation list, focused on event handling.
Accessibility
Can be invoked by any code that has access to it.
Controlled invocation, only the defining class can invoke.
Design Purpose
Provide a method of passing methods as parameters, supporting dynamic method invocation.
Facilitate a publish-subscribe model for loose coupling in event-driven design.
ADVERTISEMENT
Delegates in C# and Events in C# Definitions
Delegates in C#
A delegate is a reference type that can hold references to methods with a particular parameter list and return type.
Delegate void DisplayMessage(string message);
Events in C#
Events provide a mechanism to implement the Observer pattern in .NET, enabling loose coupling between the publisher and subscriber.
Button.Click += new EventHandler(this.OnButtonClick);
Delegates in C#
Delegates in C# enable the construction of flexible and reusable code by allowing methods to be passed as parameters.
Calculate(delegate(int x, int y) { return x + y; });
Events in C#
Events are used to signal state changes or occurrences of specific actions in a class.
Public event Action ProgressUpdated;
Delegates in C#
Delegates in C# serve as the foundation for event handling and asynchronous programming.
BeginInvoke(new Action(() => ProcessData(data)));
Events in C#
Events in C# are a way for a class to broadcast notifications to subscribed clients.
Public event EventHandler DataProcessed;
Delegates in C#
Delegates provide a way to implement callback mechanisms in C#.
Array.Sort(numbers, delegate(int x, int y) { return x.CompareTo(y); });
Events in C#
Events in C# are special types of multicast delegates that can only be invoked from within the class that declares them.
Protected virtual void OnFileDownloaded(EventArgs e) { FileDownloaded?.Invoke(this, e); }
Delegates in C#
Delegates can be multicast, meaning they can invoke multiple methods in a single call.
Operation += Add; operation += Multiply;
Events in C#
Events in C# encapsulate a delegate, allowing external classes to subscribe to or unsubscribe from event notifications.
Timer.Elapsed += new ElapsedEventHandler(OnTimedEvent);
FAQs
How do events differ from delegates in C#?
Events are a specialized form of delegates that provide a more controlled way of subscribing to and handling notifications.
How are delegates used in C#?
Delegates are used to pass methods as arguments, for callbacks, and for dynamic invocation of methods.
Can a C# delegate reference multiple methods?
Yes, C# delegates can be multicast, meaning they can reference and invoke multiple methods.
What is the purpose of the EventHandler delegate in C#?
EventHandler is a predefined delegate type used for general-purpose event handling in C#.
What is an event in C#?
An event is a special type of delegate used for implementing event-driven programming, allowing objects to notify others about state changes or occurrences.
What is a delegate in C#?
A delegate is a type-safe function pointer that can reference and invoke methods with a specific signature in C#.
Can events in C# be directly invoked from outside the class?
No, events are typically invoked only within the class that declares them, ensuring encapsulation.
Are delegates in C# type-safe?
Yes, delegates are type-safe, meaning they enforce the correct method signature and return type.
What is delegate chaining in C#?
Delegate chaining refers to adding multiple methods to a delegate's invocation list, allowing them to be called in sequence.
Can we unsubscribe from a C# event?
Yes, you can unsubscribe from an event using the -= operator to remove the method from the event's invocation list.
How do events support loose coupling in C#?
Events enable objects to signal information without needing to know the specifics of the subscriber's methods, thus supporting loose coupling.
Can a C# delegate reference a method of any return type?
A delegate can reference methods with any return type, as long as the return type matches the delegate's definition.
What is an anonymous delegate in C#?
An anonymous delegate is a delegate with no name, defined using inline code, often used for simple callback scenarios.
How does the event keyword in C# protect the delegate?
The event keyword restricts external objects from directly invoking or resetting the delegate, enforcing encapsulation.
Can events in C# be static?
Yes, events can be static, allowing them to be associated with the class itself rather than an instance.
How do you define a custom delegate in C#?
A custom delegate is defined using the delegate keyword followed by the desired method signature.
Why use delegates in C#?
Delegates provide flexibility in program design, allowing methods to be dynamically invoked and passed around like objects.
Are events in C# multicast like delegates?
Yes, events can reference multiple subscriber methods, similar to multicast delegates.
How does event subscription work in C#?
Objects subscribe to an event by appending their method to the event's invocation list, usually using the += operator.
What is delegate covariance and contravariance in C#?
Covariance and contravariance in delegates allow for flexibility with return types and parameter types, respectively, in certain situations.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
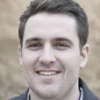