Boxing in C# vs. Unboxing in C#: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 7, 2024
Boxing in C# is converting a value type to an object type, while unboxing is converting an object type back to a value type.

Key Differences
Boxing in C# is the process of converting a value type (like int or double) into an object type. This is done implicitly by the C# runtime, encapsulating the value type inside an object on the heap. Unboxing, conversely, is the explicit conversion of an object type back to a value type. It extracts the value type from the object, reverting it to its original stack-based form.
When boxing, a value type is wrapped within a System.Object, which involves a memory allocation on the heap. This can lead to performance overhead due to the additional memory management required. Unboxing, on the other hand, requires the object to be explicitly cast back to the original value type, which involves checking the compatibility of the object with the value type, adding a small computational overhead.
Boxing is often used in scenarios where value types need to be passed to methods expecting an object, or stored in data structures that hold objects. It provides a way to treat value types as reference types. In contrast, unboxing is used when the stored value needs to be retrieved in its original type, typically when interacting with generic collections or APIs that return objects.
A key aspect of boxing is that it creates a new object, leading to a disconnection between the original value type and the boxed object. Changes made to the boxed object do not affect the original value. Conversely, when unboxing, the extracted value is a direct copy of the data in the object, allowing modifications to the unboxed value without affecting the boxed object.
Boxing in C# can be seen as a type safety feature, ensuring that value types can be used in a polymorphic way with reference types. However, unboxing requires careful handling to avoid errors such as InvalidCastException, which occur if the object is not of the expected value type.
ADVERTISEMENT
Comparison Chart
Process
Conversion from value to object type
Conversion from object to value type
Memory Allocation
Allocates memory on the heap
No new memory allocation
Usage Scenario
When value types need to be treated as reference types
When retrieving original value type from an object
Type Safety
Implicit conversion; type-safe
Requires explicit casting; prone to casting errors
Performance Impact
Can increase due to heap allocation
Can decrease due to type-checking overhead
ADVERTISEMENT
Boxing in C# and Unboxing in C# Definitions
Boxing in C#
Boxing is wrapping a value type in an object type in C#.
Int i = 123; object o = i; // Boxing
Unboxing in C#
Unboxing in C# is casting an object back to a specific value type.
Object obj = 3.14; double d = (double)obj; // Unboxing
Boxing in C#
In boxing, a value type is encapsulated within a System.Object.
Object box = 10; // Boxing an integer
Unboxing in C#
Unboxing is explicit conversion from object to a struct or primitive.
Object obj = 'x'; char ch = (char)obj; // Unboxing
Boxing in C#
Boxing in C# occurs when a value type is assigned to an object variable.
Double d = 3.14; object obj = d; // Boxing
Unboxing in C#
Unboxing extracts the value type from an object in C#.
Object o = 10; int i = (int)o; // Unboxing
Boxing in C#
Boxing converts value types to object, enabling polymorphic behavior.
ArrayList list = new ArrayList(); list.Add(42); // Boxing
Unboxing in C#
Unboxing converts an object to its original value type form.
Object box = 42; int number = (int)box; // Unboxing
Boxing in C#
Boxing is implicit conversion of structs or primitives to object type.
Char ch = 'x'; object obj = ch; // Boxing
Unboxing in C#
In unboxing, a value type is retrieved from a boxed object.
ArrayList list = new ArrayList(); list.Add(10); int val = (int)list[0]; // Unboxing
FAQs
Why is boxing used in C#?
To treat value types as reference types for polymorphism or storing in collections.
What is boxing in C#?
Boxing is converting a value type to an object type in C#.
Does boxing in C# affect performance?
Yes, it involves heap allocation and can reduce performance.
When is unboxing necessary in C#?
When retrieving the original value type stored in an object.
What is unboxing in C#?
Unboxing is converting an object type back to a value type in C#.
Is boxing in C# type-safe?
Yes, boxing is type-safe as it converts to System.Object.
Does unboxing require explicit casting in C#?
Yes, unboxing always requires explicit casting.
Can unboxing change the original boxed object in C#?
No, unboxing creates a copy of the value, leaving the boxed object unchanged.
How can unboxing errors be avoided in C#?
By ensuring the object's type matches the expected value type.
Can all objects be unboxed in C#?
Only objects that were previously boxed from a value type.
Can boxing occur implicitly in C#?
Yes, it often happens implicitly when assigning value types to object variables.
Can unboxing lead to InvalidCastException in C#?
Yes, if the object's type doesn't match the type being cast to.
Is unboxing in C# error-prone?
Yes, incorrect casting during unboxing can lead to runtime errors.
What happens to the original value during boxing in C#?
It gets copied into a new object on the heap, disconnecting from the original.
How does unboxing work with generics in C#?
Generics can reduce the need for unboxing by handling specific types.
Why is boxing necessary in non-generic collections in C#?
Because non-generic collections hold items as objects, requiring boxing for value types.
Is it possible to avoid boxing in C#?
Yes, by using generics or avoiding assigning value types to object types.
How does the .NET runtime handle boxing and unboxing?
It handles them automatically but requires careful programming to avoid performance issues.
What types of data can be boxed in C#?
Any value type, including primitives and structs, can be boxed.
What is the impact of boxing on memory in C#?
It increases memory usage due to additional object creation on the heap.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
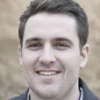
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
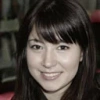