Iterator in Java vs. ListIterator in Java: What's the Difference?

Edited by Aimie Carlson || By Janet White || Published on February 13, 2024
An iterator in Java is a universal iterator for traversing through collections, whereas a ListIterator is exclusive to lists and allows bidirectional traversal and element modification.
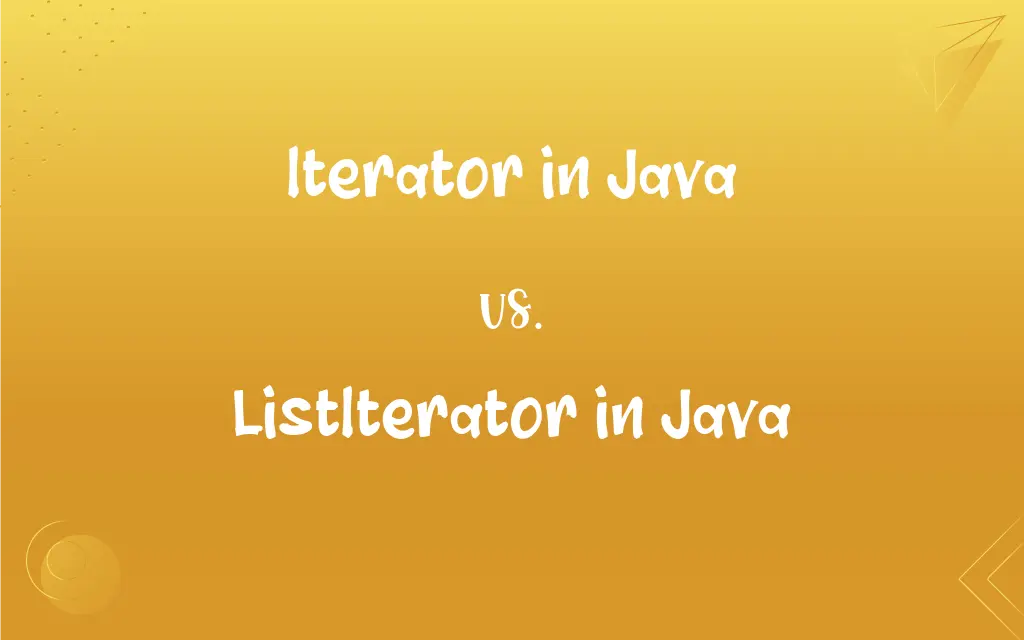
Key Differences
In Java, an iterator is a universal tool to iterate through collections like lists, sets, and more, providing forward traversal using next(). ListIterator, specific to List implementations, offers more functionality, including bidirectional traversal, with methods like previous() and next().
An iterator in Java allows for the removal of elements from a collection during iteration using remove(). ListIterator goes further by providing methods to modify the list during iteration, such as add() and set(), in addition to removal.
Java’s iterator does not provide any method to obtain the current position index during iteration. In contrast, ListIterator offers methods like nextIndex() and previousIndex() to retrieve the index of elements being traversed.
The iterator in Java is used when only forward traversal or removal is needed in various collection types. ListIterator is chosen for more complex operations on lists, like bidirectional traversal and modification of elements.
Iterator is a more generic and flexible tool for collection traversal, while ListIterator provides specialized functionalities tailored for list structures, making it more suitable for specific scenarios requiring detailed list manipulation.
ADVERTISEMENT
Comparison Chart
Direction of Traversal
Forward only
Bidirectional (forward and backward)
Collection Compatibility
All collections
Lists only
Modification Methods
Removal only (remove())
Addition (add()), modification (set()), and removal (remove())
Index Accessibility
No index information available
Provides index information (nextIndex(), previousIndex())
Use Cases
General purpose for all collections
Specific to lists, for detailed manipulation
ADVERTISEMENT
Iterator in Java and ListIterator in Java Definitions
Iterator in Java
Iterator is a basic tool for collection traversal in Java.
Iterator bookIterator = library.iterator(); while (bookIterator.hasNext()) { bookIterator.next().display(); }
ListIterator in Java
ListIterator allows adding, removing, and replacing elements during iteration.
ListIterator li = digits.listIterator(); while (li.hasNext()) { li.set(li.next() + 1); }
Iterator in Java
Iterator does not support element addition or replacement.
Iterator i = prices.iterator(); while (i.hasNext()) { System.out.println(i.next()); }
ListIterator in Java
ListIterator is an interface for bidirectional iteration over list elements.
ListIterator listIterator = names.listIterator(); while (listIterator.hasNext()) { System.out.println(listIterator.next()); }
Iterator in Java
Iterator allows safe removal of elements during iteration.
While (it.hasNext()) { if (it.next().equals(Apple)) { it.remove(); } }
ListIterator in Java
ListIterator provides methods to obtain the next and previous indices.
While (listIterator.hasNext()) { System.out.println(Index: + listIterator.nextIndex() + , Value: + listIterator.next()); }
Iterator in Java
Iterator is an interface for iterating over collection elements.
Iterator it = list.iterator(); while (it.hasNext()) { System.out.println(it.next()); }
ListIterator in Java
ListIterator supports both forward and backward traversal.
While (listIterator.hasPrevious()) { System.out.println(listIterator.previous()); }
Iterator in Java
Iterator provides a way to traverse collections in a forward direction.
For (Iterator i = numbers.iterator(); i.hasNext();) { System.out.println(i.next()); }
ListIterator in Java
ListIterator is specific to List implementations in Java.
ListIterator bookListIterator = bookList.listIterator(); while (bookListIterator.hasNext()) { bookListIterator.next().display(); }
FAQs
Can ListIterator add elements to a list during iteration?
Yes, ListIterator can add elements to a list while iterating.
Is Iterator compatible with all collection types in Java?
Yes, Iterator works with all Java collection types.
Can ListIterator obtain the index of elements?
Yes, ListIterator provides methods to get the next and previous indices.
When should you use an iterator over a ListIterator?
Use an iterator for simple forward traversal across any collection type.
Does Iterator allow element modification?
Iterator only allows removal of elements, not addition or replacement.
What is an iterator in Java?
An iterator is an interface for iterating over elements in Java collections.
Can ListIterator replace an element during iteration?
Yes, ListIterator can replace elements using its set() method.
What happens if you modify a collection while iterating with an Iterator?
Modifying a collection other than through the iterator's own remove() method results in a ConcurrentModificationException.
How does Iterator handle element removal?
Iterator safely removes elements using its remove() method.
What is a ListIterator in Java?
A ListIterator is an interface for bidirectional iteration over list elements in Java.
Can ListIterator traverse in both directions?
Yes, ListIterator can traverse both forwards and backwards.
Does Iterator support backward traversal?
No, Iterator only supports forward traversal.
Are there any collections that do not support ListIterator?
Yes, non-list collections like Set and Queue do not support ListIterator.
Is it possible to iterate in reverse order using Iterator?
No, reverse iteration is not possible with a standard Iterator.
How do ListIterator's add() and set() methods differ?
add() inserts a new element, while set() replaces the last element returned by next() or previous().
Does using an Iterator require importing any package?
Yes, both Iterator and ListIterator require importing java.util.
Can Iterator and ListIterator be used in a foreach loop?
No, they are used in while loops for iteration, not in foreach loops.
Can ListIterator start from a specific position in the list?
Yes, ListIterator can start from a specific index using list.listIterator(int index).
Can you remove elements with ListIterator?
Yes, ListIterator can remove elements during iteration.
Are Iterator and ListIterator fail-fast?
Yes, both are fail-fast and throw ConcurrentModificationException if the collection is modified during iteration, except through their own methods.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
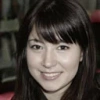