Final in Java vs. Finalize in Java: What's the Difference?

Edited by Aimie Carlson || By Janet White || Published on March 25, 2024
In Java, final is a keyword to declare constants and prevent inheritance or overriding, whereas finalize is a method for cleanup before garbage collection.

Key Differences
The final keyword in Java is used to declare variables, methods, and classes that cannot be modified further. It indicates that a variable is a constant, a method cannot be overridden, or a class cannot be subclassed. In contrast, finalize is a method used to perform cleanup operations on objects just before they are garbage collected.
When final is used with a variable, the value of this variable cannot be changed once it is initialized. With methods, final prevents them from being overridden in subclasses. When applied to a class, it prevents the class from being subclassed. On the other hand, the finalize method is called by the garbage collector on an object when garbage collection determines that there are no more references to the object.
The concept of final is mainly related to variable immutability, method overriding, and class inheritance. It is a design decision at the coding level. Finalize, however, deals with memory management and cleanup, and it is part of the Java runtime's garbage collection mechanism.
Using the final keyword can make Java code more robust by preventing accidental changes to variables or class structures. Conversely, the finalize method is generally used to release system resources or perform other cleanup before the object is collected by the garbage collector.
The final keyword is a fundamental part of Java's type system and its inheritance model. Finalize is part of Java's object lifecycle and garbage collection system. It is recommended to use final judiciously for clarity and to avoid finalize for manual memory management due to its unpredictability and performance impacts.
ADVERTISEMENT
Comparison Chart
Purpose
To create constants, prevent method overriding, and subclassing
To perform cleanup operations before garbage collection
Usage
With variables, methods, and classes
As a method in an object's class
Function
Provides immutability and security in code
Helps in cleaning up resources before object disposal
Impact on Design
Affects class design and inheritance
Linked to object lifecycle and memory management
Best Practice
Used for creating immutable objects and safe coding
Generally avoided due to unpredictability and potential performance issues
ADVERTISEMENT
Final in Java and Finalize in Java Definitions
Final in Java
Prevents a method from being overridden.
Public final void display() { /* Code */ }
Finalize in Java
Used for cleaning up resources before object disposal.
Public void finalize() { /* Release resources */ }
Final in Java
Prevents a class from being subclassed.
Public final class MyImmutableClass { /* Code */ }
Finalize in Java
A method to perform final actions before an object is garbage collected.
Protected void finalize() { /* Close file streams */ }
Final in Java
Declares a variable as constant.
Final int MAX_HEIGHT = 200;
Finalize in Java
A method called by the garbage collector.
Protected void finalize() { /* Cleanup code */ }
Final in Java
Signifies immutability in variables.
Final String USERNAME = user123;
Finalize in Java
A method invoked at the discretion of the garbage collector.
Protected void finalize() { /* Clear references */ }
Final in Java
Establishes fixed behavior for methods and classes.
Final void calculateTotal() { /* Code */ }
Finalize in Java
Part of the object cleanup process in Java's memory management.
Protected void finalize() { /* Free native resources */ }
FAQs
Is final a keyword or method in Java?
final is a keyword in Java.
Can a final class be extended?
No, a final class cannot be subclassed.
What is the purpose of the finalize method?
To perform cleanup operations before an object is garbage collected.
Can final variables be modified?
No, final variables cannot be modified once initialized.
What happens if you try to override a final method?
The compiler will throw an error.
Is it mandatory to initialize a final variable at declaration?
Not mandatory, but it must be initialized before use.
Can the finalize method be overridden?
Yes, finalize can be overridden.
Can finalize be used for memory deallocation?
No, memory deallocation is handled by the garbage collector.
What's a major drawback of using finalize?
It may cause delays in resource release and is unpredictable.
Is finalize automatically called by Java runtime?
Yes, it's called by the garbage collector.
Does a final variable improve performance?
It can, as it helps the compiler make optimizations.
Can a final method be inherited?
Yes, but it cannot be overridden.
Can final be applied to method parameters?
Yes, to prevent changing the reference within the method.
How is final used in anonymous classes?
To use variables from the enclosing context in the anonymous class.
Is it good practice to rely on finalize for resource management?
No, it's not recommended due to its unpredictability.
What's a common use case for final?
To create immutable objects and constant values.
Does finalize guarantee timely resource release?
No, its execution time is uncertain.
Are all final variables constants?
Yes, in the context of their initialized values.
Can finalize be called explicitly in code?
Yes, but it's not a standard practice.
Is it better to use try-with-resources or finalize?
Try-with-resources is preferable for better resource management.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
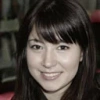