Checked Exceptions in Java vs. Unchecked Exceptions in Java: What's the Difference?

Edited by Aimie Carlson || By Janet White || Published on February 25, 2024
Checked exceptions are those that Java compiler forces to catch or declare, while unchecked exceptions are runtime errors not checked at compile-time.
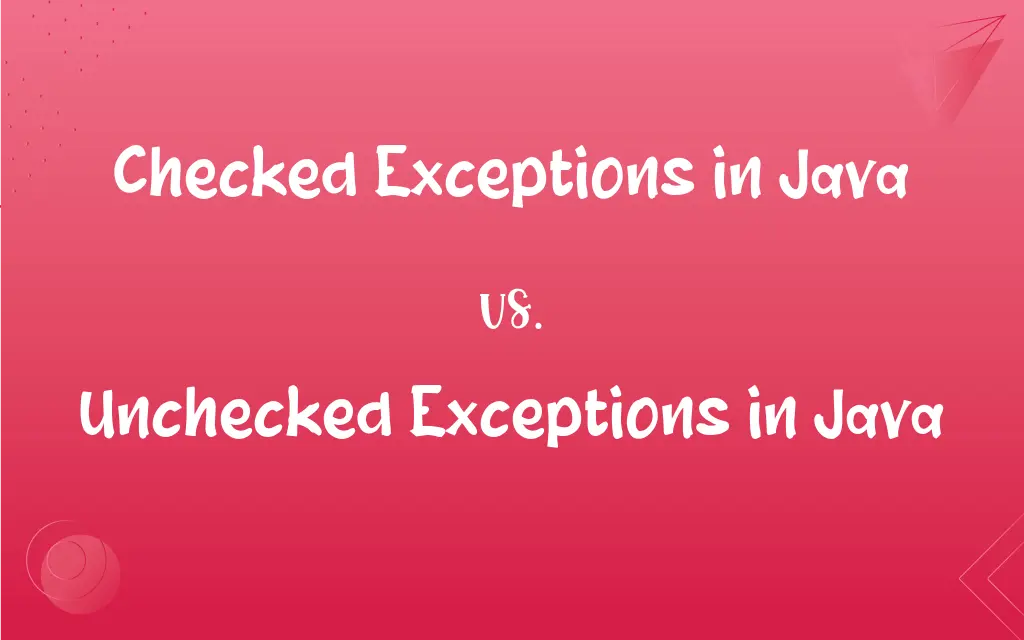
Key Differences
Checked Exceptions in Java exceptions are checked at compile-time, meaning the compiler ensures that they are either caught in a try-catch block or declared in the method's throws clause. In contrast, unchecked exceptions occur during runtime and are not required to be handled or declared in the program.
Checked Exceptions in Java are subclasses of Exception but not of RuntimeException. They represent conditions that a reasonable application might want to catch. Unchecked Exceptions in Java are subclasses of RuntimeException, and they represent problems that are a result of a programming error, like accessing a null pointer.
In Checked Exceptions, the programmer is forced to handle these exceptions, making the code more robust by explicitly handling error conditions. Since Unchecked Exceptions are not checked at compile-time, they can potentially lead to program crashes if not properly accounted for.
Checked Exceptions encourage error handling and increase the reliability of the application. Unchecked Exceptions exceptions give the programmer the freedom to decide whether to handle them, based on the specific scenario.
Comparison Chart
Checked at
Compile-time
Runtime
ADVERTISEMENT
Subclass of
Exception (excluding RuntimeException)
RuntimeException
Handling
Mandatory (try-catch or throws)
Optional
Example
IOException, SQLException
NullPointerException, ArithmeticException
Purpose
Handling recoverable errors
Indicating programming errors
Checked Exceptions in Java and Unchecked Exceptions in Java Definitions
Checked Exceptions in Java
Exceptions checked at compile time by the Java compiler.
Public void readFile() throws FileNotFoundException { // code to read file }
ADVERTISEMENT
Unchecked Exceptions in Java
Optional to handle, giving flexibility to programmers.
Int[] arr = new int[5]; arr[10] = 100; // ArrayIndexOutOfBoundsException
Checked Exceptions in Java
Representing recoverable conditions and prompting proper handling.
Try { connection.connect(); } catch (SQLException e) { // handle SQLException }
Unchecked Exceptions in Java
Subclasses of RuntimeException.
List list = null; list.add(test); // NullPointerException
Checked Exceptions in Java
Exceptions that must be either caught or declared in a method.
Try { file.read(); } catch (IOException e) { // handle IOException }
Unchecked Exceptions in Java
Often resulting from programming errors like bad casting or invalid array access.
String s = null; s.length(); // causes NullPointerException
Checked Exceptions in Java
Enforcing error handling to improve software reliability.
Public void saveData() throws IOException { // code to save data }
Unchecked Exceptions in Java
Runtime exceptions that are not checked at compile time.
Int a = 5 / 0; // leads to ArithmeticException
Checked Exceptions in Java
Non-runtime exceptions that signal issues like IO errors or problems with external resources.
Try { new FileInputStream(file); } catch (FileNotFoundException e) { // handle exception }
Unchecked Exceptions in Java
Indicative of bugs in program logic that need fixing rather than catching.
Object x = new Integer(0); System.out.println((String)x); // ClassCastException
FAQs
What are checked exceptions in Java?
Exceptions that the compiler checks for during the compile time.
Are unchecked exceptions part of the Java language specification?
Yes, they are part of Java's exception handling model.
How do unchecked exceptions typically arise?
From programming errors like null pointer access or illegal argument passing.
What are unchecked exceptions in Java?
Exceptions that occur at runtime and are not checked by the compiler.
How do checked exceptions improve software quality?
By enforcing error handling at compile time, leading to more robust code.
Can unchecked exceptions be manually caught in Java?
Yes, they can be caught using try-catch blocks, though it's not required.
What is a common example of a checked exception?
IOException is a common example.
Why are unchecked exceptions not checked by the Java compiler?
They often result from programming mistakes that should be fixed rather than handled.
Why must checked exceptions be handled in Java?
To ensure robust error handling and avoid compile-time errors.
Do checked exceptions need to be declared in a method's signature?
Yes, using the throws keyword.
Are all subclasses of Exception checked exceptions?
No, subclasses of RuntimeException are unchecked.
Is it good practice to throw unchecked exceptions deliberately?
Typically no, as they indicate programming errors and should be fixed rather than caught.
Can checked exceptions be avoided with design changes?
Yes, by using alternative error-handling strategies like return values.
What is a common example of an unchecked exception?
NullPointerException is a typical unchecked exception.
Are unchecked exceptions recoverable?
While they can be caught and handled, they often indicate serious programming flaws.
Is it mandatory to use try-catch for unchecked exceptions?
No, it's optional.
Should business exceptions be checked or unchecked?
It depends on the application's design, but they are often checked to enforce handling.
Does handling unchecked exceptions make a program more robust?
Catching and handling them can make the program more robust, but it's often better to fix the underlying programming error.
Can checked exceptions be ignored in Java?
No, they must be handled or declared.
Can checked exceptions be converted to unchecked exceptions?
Yes, by wrapping them in a RuntimeException.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
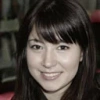