ArrayList in Java vs. Vector in Java: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 24, 2024
ArrayList in Java is a resizable array implementation, while Vector is a synchronized, resizable array.

Key Differences
ArrayList in Java is part of the Collections framework and represents a dynamic array that can grow or shrink in size. It provides fast access and is not synchronized, making it unsuitable for multi-threaded environments. Vector, on the other hand, is a legacy class from the early versions of Java and is synchronized, meaning it is thread-safe but slower due to the overhead of synchronization.
In terms of performance, ArrayList is generally faster and more efficient as it is non-synchronized. It's preferred in single-threaded or read-intensive operations where synchronization is not a concern. Vector, however, is suitable for multi-threaded operations where thread safety is necessary, although its use has diminished in favor of newer concurrent collections.
ArrayList provides better control over capacity, with its capacity increasing dynamically. It increases its size by 50% of the array size when it runs out of space. In contrast, Vector defaults to doubling the size of its array when it needs to grow, which can be memory-inefficient.
When it comes to iterating over elements, ArrayList provides iterator and listIterator methods which are fail-fast. This means they can throw a ConcurrentModificationException if the list is structurally modified during iteration. Vector also offers an Enumeration method in addition to Iterators, providing a more traditional way of traversing its elements.
ArrayList is a part of the Java Collections Framework and is more commonly used in modern Java applications. It's more flexible and offers better performance in most scenarios. Vector, while still used, is considered somewhat outdated and is generally recommended only in specific, thread-safe applications.
ADVERTISEMENT
Comparison Chart
Synchronization
Non-synchronized
Synchronized
Performance
Faster due to no synchronization
Slower due to synchronization
Capacity Increase
Increases by 50% of its size
Doubles its size
Thread Safety
Not thread-safe
Thread-safe
Iteration Methods
Iterator and listIterator (fail-fast)
Iterator, listIterator, Enumeration
ADVERTISEMENT
ArrayList in Java and Vector in Java Definitions
ArrayList in Java
ArrayList allows duplicate elements and maintains insertion order.
Colors.add(Red); colors.add(Blue);
Vector in Java
Vector allows duplicate elements and maintains insertion order.
Animals.add(Dog); animals.add(Cat);
ArrayList in Java
ArrayList provides random access to its elements.
String firstColor = colors.get(0);
Vector in Java
Vector provides both Enumeration and Iterator for traversing its elements.
Enumeration enum = animals.elements();
ArrayList in Java
ArrayList is a resizable-array implementation of the List interface.
ArrayList colors = new ArrayList<>();
Vector in Java
Vector provides thread safety through its synchronized methods.
Synchronized(animals) { animals.add(Lion); }
ArrayList in Java
ArrayList is not synchronized and is suitable for non-threaded applications.
ArrayList numbers = new ArrayList<>(Arrays.asList(1, 2, 3));
Vector in Java
Vector is a synchronized, resizable-array implementation of the List interface.
Vector animals = new Vector<>();
ArrayList in Java
ArrayList can dynamically grow and shrink as needed.
Colors.remove(Blue);
Vector in Java
Vector doubles the size of its array when it runs out of space.
Vector numbers = new Vector<>(10);
FAQs
How does ArrayList manage its capacity?
It increases its capacity by 50% when it runs out of space.
Can ArrayList contain duplicate elements?
Yes, ArrayList allows duplicates.
What is Vector in Java?
Vector is a synchronized, resizable array implementation.
How does Vector increase its capacity?
Vector doubles its size when more space is needed.
Is ArrayList thread-safe?
No, it's not inherently thread-safe.
What is ArrayList in Java?
It's a resizable array implementation from the Java Collections framework.
When should I use ArrayList?
Use it in single-threaded applications or when synchronization isn't needed.
How does iteration differ between ArrayList and Vector?
ArrayList uses iterators which are fail-fast; Vector also offers Enumerations.
How do I choose between ArrayList and Vector?
Consider thread safety needs and performance requirements.
When is using Vector appropriate?
Use Vector in multi-threaded scenarios where synchronization is important.
Can I convert a Vector to an ArrayList?
Yes, using new ArrayList<>(vector).
Can I store elements of different types in ArrayList or Vector?
Yes, if they are defined to store Object or a common superclass.
Are there alternatives to Vector for thread-safe operations?
Yes, like CopyOnWriteArrayList, or Collections.synchronizedList().
Does Vector allow duplicates?
Yes, like ArrayList, it allows duplicates.
Is Vector part of the Java Collections Framework?
Yes, it is, but it predates the modern Collections framework.
Can I create a synchronized ArrayList?
Yes, by wrapping it with Collections.synchronizedList().
Do ArrayList and Vector maintain order of elements?
Yes, both maintain the insertion order of elements.
How does ArrayList handle thread safety?
It doesn't; external synchronization is needed for thread safety.
Is Vector slower than ArrayList?
Generally, yes, due to its synchronized methods.
Are ArrayList and Vector interchangeable?
They can be, but they have different performance and threading characteristics.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
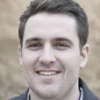
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
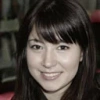