Virtual Function vs. Pure Virtual Function: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 4, 2024
Virtual function in C++ is a member function which can be overridden in derived classes, while a pure virtual function is a virtual function with no implementation, making the class abstract.

Key Differences
A virtual function in C++ is a function declared in a base class using the keyword 'virtual' and is intended to be overridden in derived classes. It facilitates runtime polymorphism, allowing the program to decide which function to invoke at runtime. On the other hand, a pure virtual function, also declared in the base class, has no implementation in that class and is declared by assigning it to 0. It serves to create an abstract class, which cannot be instantiated and mandates derived classes to provide an implementation for this function.
In the context of object-oriented programming, a virtual function allows derived classes to have different implementations of the same function. This concept enhances flexibility and reusability of code. A pure virtual function, however, is used when the base class has a function that must be implemented, but the base class does not provide a meaningful implementation. The pure virtual function is thus a way to enforce a contract for derived classes to implement specific functionality.
Virtual functions in C++ are used to achieve dynamic polymorphism. They allow the program to call a function based on the type of the object pointed to by the base class pointer, rather than the type of the pointer itself. In contrast, pure virtual functions are used to create interfaces or abstract classes in C++. By declaring at least one pure virtual function in a class, it becomes an abstract class, meaning it cannot be instantiated directly.
The virtual function mechanism is crucial for achieving run-time polymorphism in C++. When a virtual function is called through a pointer or a reference to the base class, C++ determines which derived class's function to call at runtime. Pure virtual functions, by their nature, cannot be called directly since they lack implementation in the base class. Their primary purpose is to ensure that the derived classes provide a specific implementation of these functions.
Virtual functions in C++ are often implemented with a default behavior in the base class, which can be overridden in the derived class. This allows classes to share a common interface but have different behaviors. Pure virtual functions do not have this default behavior in the base class. Their declaration simply serves as a template for the derived classes, making it mandatory for the derived classes to override these functions.
ADVERTISEMENT
Comparison Chart
Definition
A function that can be overridden in derived classes.
A function without an implementation in the base class.
Purpose
To allow different implementations in derived classes.
To create abstract classes and enforce implementation in derived classes.
Implementation in Base Class
Can have an implementation.
Has no implementation (assigned to 0).
Usage
To achieve runtime polymorphism.
To create interfaces and abstract classes.
Instantiation
Does not affect the instantiation of the base class.
Prevents the base class from being instantiated directly.
ADVERTISEMENT
Virtual Function and Pure Virtual Function Definitions
Virtual Function
A virtual function is a member function in a base class that can be overridden in derived classes.
In class Animal, the virtual function makeSound() allows class Dog to have its own implementation of makeSound().
Pure Virtual Function
A pure virtual function creates an abstract class when used in a base class.
With virtual void communicate() = 0 in class Animal, the Animal class becomes abstract, requiring subclasses to implement communicate().
Virtual Function
A virtual function is declared with the virtual keyword in a base class.
In class Shape, declaring virtual void draw() allows class Circle to implement its own version of draw().
Pure Virtual Function
A pure virtual function is a tool for enforcing a contract for functionality in derived classes.
Virtual void save() = 0 in class PersistentObject ensures that all subclasses like class Document must implement save().
Virtual Function
A virtual function enables runtime polymorphism in C++.
Using a pointer of type Animal to call makeSound(), the program decides at runtime whether to call Dog's or Cat's makeSound().
Pure Virtual Function
A pure virtual function is used to define an interface in C++.
Class Worker with virtual void work() = 0 makes it essential for any derived class like class Engineer to define work().
Virtual Function
A virtual function allows derived classes to have a different implementation than the base class.
Class Bird can override the virtual function fly() from class Animal to implement its specific flying behavior.
Pure Virtual Function
A pure virtual function is a virtual function with no implementation in the base class, set to 0.
Declaring virtual void draw() = 0 in class Shape forces class Circle to provide an implementation for draw().
Virtual Function
A virtual function provides a common interface for different derived classes.
Draw() can be a virtual function in class GraphicObject, allowing both class Circle and class Square to implement it differently.
Pure Virtual Function
A pure virtual function mandates that derived classes must provide their own implementation.
In class Vehicle, virtual void startEngine() = 0 requires class Car to define startEngine().
FAQs
Can a virtual function have a default implementation?
Yes, a virtual function can have a default implementation in the base class.
How do virtual functions achieve polymorphism?
By allowing derived classes to provide different implementations for the same function.
Is it mandatory to override a virtual function?
No, it is not mandatory to override a virtual function in the derived class.
Can a pure virtual function be private?
Yes, but it would limit its overriding to friend classes or functions.
Why use pure virtual functions?
To create abstract classes and enforce specific implementations in derived classes.
What is a pure virtual function?
A virtual function with no implementation in the base class, making the class abstract.
What is a virtual function?
A member function in a base class that can be overridden in derived classes.
What is an abstract class?
A class with at least one pure virtual function, intended to be a base class.
What is runtime polymorphism?
A feature in OOP where the call to an overridden function is resolved at runtime.
What happens if a pure virtual function is not overridden?
The derived class will also become abstract and cannot be instantiated.
Can we instantiate an abstract class?
No, an abstract class cannot be instantiated directly.
What is a virtual table in C++?
A mechanism used by C++ to support dynamic dispatch of virtual functions.
What is the syntax for declaring a pure virtual function?
virtual ReturnType FunctionName(parameters) = 0;
Why use virtual functions?
To enable runtime polymorphism and flexible function overriding in derived classes.
How does a pure virtual function enforce subclass behavior?
By requiring derived classes to provide specific implementations of the function.
Can a constructor be virtual?
No, constructors cannot be virtual in C++.
Can a destructor be virtual?
Yes, destructors should be virtual in base classes with derived classes.
How does a virtual function differ from a regular function?
A virtual function allows for overriding in derived classes, unlike a regular function.
Can a pure virtual function have an implementation?
Yes, a pure virtual function can have an implementation, but it's uncommon.
Does a derived class inherit the virtual nature of a function?
Yes, once a function is declared virtual in a base class, it remains virtual in all derived classes.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
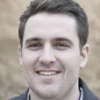
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
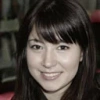