New vs. Malloc( ): What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 1, 2024
New initializes objects, calling constructors in C++; Malloc() allocates memory without initialization in C.
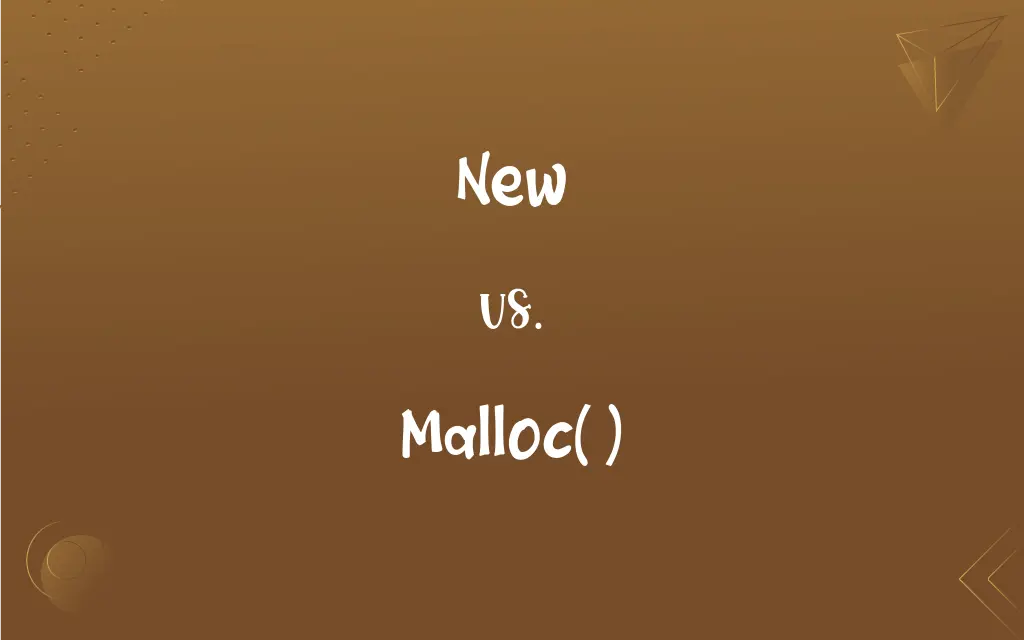
Key Differences
New in C++ not only allocates memory but also initializes the object by calling the constructor. It returns a fully typed pointer, ensuring type safety. For instance, new int allocates memory for an integer and initializes it. Malloc(), a C function, allocates a specified amount of memory and returns a void pointer to it. This pointer can then be cast to any desired type. Malloc() does not initialize the memory; it leaves it with indeterminate content.
New throws an exception when memory allocation fails, providing a robust way to handle memory allocation errors. It's part of C++'s standard exception handling mechanism. Malloc(), when failing to allocate memory, returns a null pointer. This requires manual error checking after each call to Malloc(), a practice that is error-prone and often overlooked.
New can be overloaded and customized in C++, allowing developers to define their own behavior for memory allocation and object initialization. Malloc() is a library function and cannot be overloaded. It follows a fixed behavior defined by the C standard library, providing a simple, straightforward way to allocate memory without any frills.
Comparison Chart
Language
C++
C
Initialization
Allocates and initializes
Allocates without initialization
ADVERTISEMENT
Error Handling
Throws an exception on failure
Returns NULL on failure
Return Type
Typed pointer
Void pointer (requires casting)
Customization
Can be overloaded
Cannot be overloaded
Memory Handling
Tied to object lifecycles
Pure memory allocation
Constructors
Calls constructors
Does not call constructors
ADVERTISEMENT
New and Malloc( ) Definitions
New
Can be customized in class definitions.
Void* MyClass::operator new(size_t size) { /* custom allocation */ }
Malloc( )
Allocates a block of memory without initializing.
Int* p = (int*)malloc(sizeof(int));
New
Allocates and initializes objects.
Int* p = new int(5);
Malloc( )
Returns NULL if memory cannot be allocated.
Int* p = (int*)malloc(sizeof(int)); if (!p) { /* handle error */ }
New
Allocates memory for a specific type.
Double* p = new double;
Malloc( )
Requires the size of memory to allocate.
Float* array = (float*)malloc(10 * sizeof(float));
New
Automatically calls the constructor of the class.
MyClass* obj = new MyClass();
Malloc( )
Part of the C standard library.
Void* memory = malloc(1024);
New
Throws a bad_alloc exception if memory allocation fails.
Try { int* p = new int; } catch (std::bad_alloc& e) { /* handle error */ }
Malloc( )
Returns a void pointer, requiring type casting.
Char* str = (char*)malloc(50 * sizeof(char));
Malloc( )
(computing) A subroutine in the C programming language's standard library for performing dynamic memory allocation.
Malloc( )
(computing) To allocate memory using the C programming language malloc subroutine.
FAQs
How does 'new' differ from traditional memory allocation?
'new' not only allocates memory but also initializes the memory by calling the object's constructor.
Can you give a basic example of using 'new'?
int* ptr = new int; allocates memory for an integer.
What is 'new' in C++?
'new' is a C++ operator used for dynamic memory allocation.
What happens if 'new' fails in C++?
If 'new' fails, it throws a bad_alloc exception.
Is 'new' a function or an operator?
'new' is an operator in C++.
Can 'new' be used for array allocation?
Yes, e.g., int* arr = new int[10]; allocates an array of 10 integers.
Does 'new' return a pointer?
Yes, 'new' returns a pointer to the allocated memory.
What is placement 'new'?
Placement 'new' allows allocating memory at a specified location.
How does 'malloc()' work?
'malloc()' allocates a specified number of bytes and returns a pointer to the allocated memory.
What is 'malloc()' in C?
'malloc()' is a function in C used for dynamic memory allocation.
What if 'malloc()' fails to allocate memory?
If 'malloc()' fails, it returns a NULL pointer.
Does 'malloc()' initialize the allocated memory?
No, 'malloc()' does not initialize the memory; it leaves it uninitialized.
How do you deallocate memory allocated by 'new'?
Use delete to deallocate memory allocated by 'new'.
Can 'new' initialize an object?
Yes, 'new' can initialize objects using constructors.
What header file is required for 'malloc()'?
The stdlib.h header file is required for 'malloc()'.
Can you provide a basic example of 'malloc()'?
int* ptr = (int*)malloc(sizeof(int)); allocates memory for an integer.
How do you free memory allocated by 'malloc()'?
Use free() to deallocate memory allocated by 'malloc()'.
Can 'malloc()' be used for arrays?
Yes, e.g., int* arr = (int*)malloc(10 * sizeof(int)); allocates an array of 10 integers.
How do you determine the size for 'malloc()' allocation?
The size is determined based on the size of the data type and the number of elements, calculated using sizeof().
Is 'malloc()' specific to C?
'malloc()' is primarily used in C, though it can also be used in C++.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
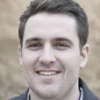
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
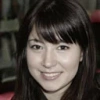