dispose() in C# vs. finalize() in C#: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 11, 2024
Dispose() is explicitly called to release unmanaged resources, while finalize() is called by the garbage collector to finalize an object before reclaiming memory.
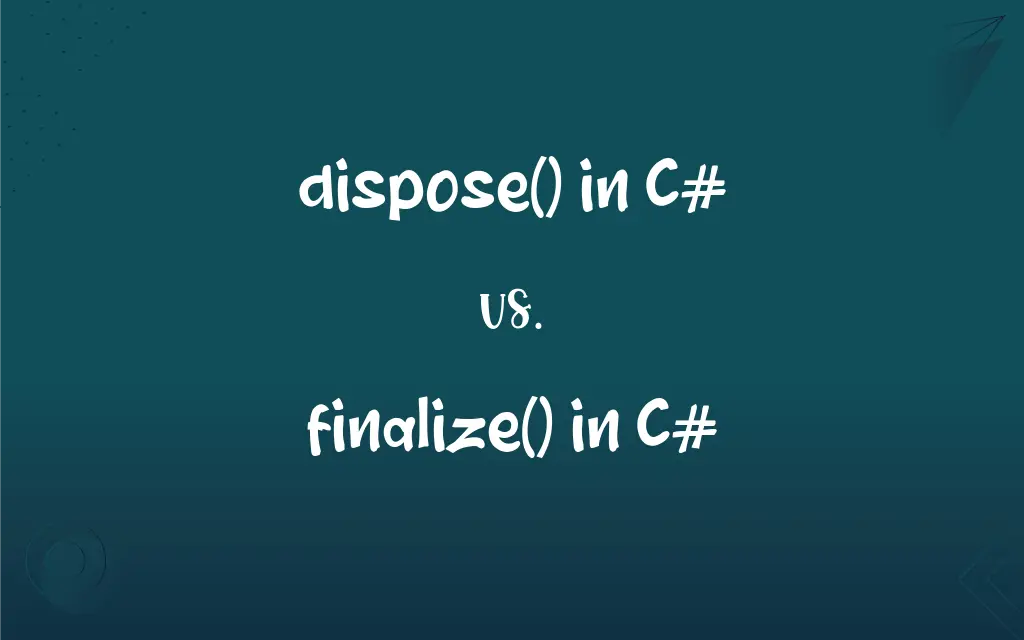
Key Differences
Dispose() is explicitly called by the developer to free unmanaged resources, like file handles or database connections. It's part of the IDisposable interface. In contrast, finalize() is invoked by the garbage collector before it reclaims the object's memory, providing a chance to release unmanaged resources if Dispose() wasn't called.
Dispose() gives developers control over when resources are released, enabling better resource management and performance tuning. finalize(), on the other hand, lacks this predictability as it's dependent on the garbage collector's schedule, which can lead to delays in resource release.
Dispose() provides deterministic disposal of resources, meaning the developer knows exactly when the resources are released. finalize() is non-deterministic; you can't predict when the garbage collector will call it.
Frequent use of finalize() can negatively impact performance since it requires additional garbage collection cycles. Using Dispose(), which is deterministic, helps in optimizing performance by timely resource management.
Dispose() is used in scenarios where timely release of resources is crucial. finalize() is a safety net to clean up resources if Dispose() wasn't called, but it's not guaranteed to run promptly or in specific scenarios like application shutdown.
ADVERTISEMENT
Comparison Chart
Invocation
Explicitly called by the developer.
Called by the garbage collector.
Resource Management
Enables deterministic management of unmanaged resources.
Non-deterministic, depends on garbage collection.
Control over Execution
Developer-controlled timing.
Garbage collector controlled timing.
Impact on Performance
Can improve performance through timely resource release.
Can degrade performance due to extra GC overhead.
Interface Implementation
Part of the IDisposable interface.
Override method in the object class.
ADVERTISEMENT
dispose() in C# and finalize() in C# Definitions
dispose() in C#
Implements IDisposable for resource management.
Public void Dispose() { /* resource cleanup code */ }
finalize() in C#
Invoked by garbage collector before object memory is reclaimed.
~MyClass() { /* cleanup code */ } // Finalize method
dispose() in C#
Manually releases unmanaged resources.
Stream.Dispose(); // Closes the file stream immediately.
finalize() in C#
Used as a last resort for resource cleanup.
// Finalize() is called if Dispose() was not used
dispose() in C#
Allows manual control over resource disposal.
Connection.Dispose(); // Manually closing a database connection.
finalize() in C#
Overridden from the base Object class.
Protected override void Finalize() { /* cleanup code */ }
dispose() in C#
Ensures timely cleanup of resources.
Using (var resource = new Resource()) { /* use resource */ } // Dispose called automatically here
finalize() in C#
Unpredictable timing for resource cleanup.
// Finalize() might not be called immediately after object is not in use
dispose() in C#
Must be explicitly called to release resources.
Graphics.Dispose(); // Explicitly releasing graphical resources.
finalize() in C#
Acts as a safety net for unmanaged resource release.
// In Finalize(), if Dispose() was not called, release resources
FAQs
Does the garbage collector call Finalize()?
Yes, Finalize() is called by the garbage collector before reclaiming an object's memory.
Is Dispose() automatically called?
No, Dispose() must be explicitly called by the developer.
Can Dispose() improve application performance?
Yes, Dispose() can improve performance by timely releasing unmanaged resources.
When should I use Dispose()?
Use Dispose() when you need to explicitly release unmanaged resources.
What is the role of Finalize() in C#?
Finalize() acts as a safety net to clean up resources if Dispose() wasn’t called.
Can Finalize() cause performance issues?
Yes, because Finalize() requires extra garbage collection cycles.
Is Finalize() deterministic?
No, Finalize() is non-deterministic as its timing depends on the garbage collector.
Can I call Dispose() multiple times?
Dispose() should be designed to allow multiple calls without throwing exceptions.
Is Dispose() part of the garbage collection process?
No, Dispose() is not part of garbage collection; it's manually invoked.
Is it good practice to rely on Finalize() for cleanup?
No, relying on Finalize() is not recommended due to its unpredictability.
Should I always implement Dispose()?
Implement Dispose() in classes that use unmanaged resources.
Is Finalize() necessary if I use Dispose()?
Finalize() acts as a backup if Dispose() is not called, but it's not necessary if Dispose() is properly used.
Can Dispose() release managed resources?
Yes, Dispose() can release both managed and unmanaged resources.
Can I override Dispose()?
Yes, you can and should override Dispose() when implementing IDisposable.
Is Finalize() called after Dispose()?
No, if Dispose() is properly implemented, Finalize() should not be called, especially if GC.SuppressFinalize() is used.
How does using() relate to Dispose()?
The using statement ensures Dispose() is called automatically.
Should Finalize() call Dispose()?
Yes, Finalize() should call Dispose(false) to release unmanaged resources.
What happens if Finalize() is not overridden?
If not overridden, the base class Finalize() or no action will be taken.
When is Finalize() typically called?
Finalize() is typically called when an object is no longer accessible.
How does GC.SuppressFinalize() relate to Dispose()?
GC.SuppressFinalize() prevents Finalize() from being called, useful after Dispose() is called.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
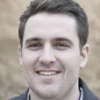
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
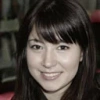