Copy Constructor in C++ vs. Assignment Operator in C++: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 20, 2024
The copy constructor in C++ initializes a new object as a copy of an existing object, while the assignment operator assigns values from one existing object to another.

Key Differences
The copy constructor in C++ is used to create a new object as a copy of an existing object. The assignment operator, on the other hand, is used to copy values from one already existing object to another already existing object.
A copy constructor is called when a new object is created from another object, ensuring a copy of the object is made. In contrast, the assignment operator is invoked when an object is assigned the value of another object, after both objects have been created.
Copy constructors initialize new objects, and they are often called implicitly, like when passing objects by value. Assignment operators are used for assigning values between objects and are called explicitly or implicitly when an object is assigned to another.
In the copy constructor, the object itself does not exist yet and is being created. With the assignment operator, both objects involved in the operation already exist, and their states can be modified.
The copy constructor plays a critical role in the creation of temporary objects and in passing and returning objects by value. The assignment operator is crucial for changing the contents of already existing objects, especially when dealing with dynamic memory.
ADVERTISEMENT
Comparison Chart
Purpose
Initializes a new object as a copy of another object.
Assigns values from one existing object to another.
Invocation Time
Called when a new object is created.
Called when an existing object is assigned a new value.
Object State
Creates a new object with its own state.
Modifies the state of an existing object.
Typical Use
Used in object initialization and copying.
Used in object reassignment and updating.
Role in Object Lifecycle
Critical in the creation phase of an object's lifecycle.
Important in the modification phase of an object's lifecycle.
ADVERTISEMENT
Copy Constructor in C++ and Assignment Operator in C++ Definitions
Copy Constructor in C++
"Creates a new object as a copy of an existing object."
MyClass obj3 = obj1; // Copy constructor called
Assignment Operator in C++
"Invoked when an object is assigned to another existing object."
Obj5 = MyClass(); // Assignment operator called
Copy Constructor in C++
"Automatically called in pass-by-value and return-by-value scenarios."
MyClass function(MyClass obj); // Copy constructor called when obj is passed by value
Assignment Operator in C++
"Allows updating the state of an existing object."
Obj3 = obj4; // obj3's state is updated
Copy Constructor in C++
"A constructor which is invoked when a new object is created from an existing object."
MyClass obj4 = MyClass(obj1); // Copy constructor creates obj4
Assignment Operator in C++
"An operator that assigns the values of one object to another existing object."
Obj1 = obj2; // Using assignment operator
Copy Constructor in C++
"Used for deep copying of object values."
MyClass obj5(myObject); // Copy constructor ensures deep copy
Assignment Operator in C++
"Used to copy values from one object to another after initialization."
Obj3 = obj4; // obj3 now has values of obj4
Copy Constructor in C++
"A constructor that initializes an object using another object of the same class."
MyClass obj1(obj2); // Using copy constructor
Assignment Operator in C++
"Can be overloaded to provide deep copy functionality."
Obj1 = obj2; // Assignment operator ensures deep copy if overloaded
FAQs
What is a copy constructor in C++?
A copy constructor initializes a new object as a copy of another object of the same class.
Can the copy constructor be overloaded?
Yes, the copy constructor can be overloaded, but it should maintain the signature of a copy constructor.
What happens if you don't define a copy constructor?
If not defined, C++ generates a default copy constructor that performs a shallow copy.
What does the assignment operator do in C++?
The assignment operator assigns values from one existing object to another.
Is assignment operator automatically provided by C++?
Yes, C++ provides a default assignment operator if one is not explicitly defined.
Can the assignment operator return a value?
Yes, the assignment operator typically returns a reference to the assigned object.
When is a copy constructor called?
A copy constructor is called when a new object is created from an existing object.
Why is the copy constructor important for objects with dynamic memory?
It's important to avoid shallow copy issues and ensure each object has its own copy of dynamic resources.
Is it mandatory to define a copy constructor?
It's not mandatory, but it's recommended for classes that manage resources like dynamic memory.
How is the assignment operator used?
The assignment operator is used to copy values between two already existing objects.
Are there any default parameters in a copy constructor?
No, a copy constructor typically has one parameter, a reference to the object being copied.
How does the copy constructor differ from the assignment operator in terms of efficiency?
The copy constructor is often more efficient as it initializes objects directly, avoiding additional assignment overhead.
How does the assignment operator handle memory management?
The assignment operator should handle memory correctly to avoid leaks and ensure deep copy when necessary.
Why might you need to define both a copy constructor and an assignment operator?
Defining both ensures that objects are copied correctly both during initialization and assignment, particularly for classes managing resources.
Can you use the assignment operator for initialization?
No, the assignment operator is not used for initialization, that's the role of constructors.
When should you overload the assignment operator?
Overload the assignment operator for classes that require deep copying or special handling during assignment.
What is self-assignment in C++?
Self-assignment occurs when an object is assigned to itself, which should be handled in the assignment operator.
Can the copy constructor and assignment operator be private?
Yes, making them private is a way to prevent copying or assignment, useful in certain design patterns.
What is the significance of the copy constructor in pass-by-value?
In pass-by-value, the copy constructor ensures that a function's parameters are a copy of the original objects.
How does the default assignment operator handle object copying?
The default assignment operator performs a shallow copy of non-static members.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
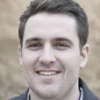
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
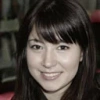