String Class in Java vs. StringBuffer Class in Java: What's the Difference?

Edited by Aimie Carlson || By Janet White || Published on March 3, 2024
In Java, String class represents immutable character strings, while StringBuffer class is used for creating mutable string sequences.
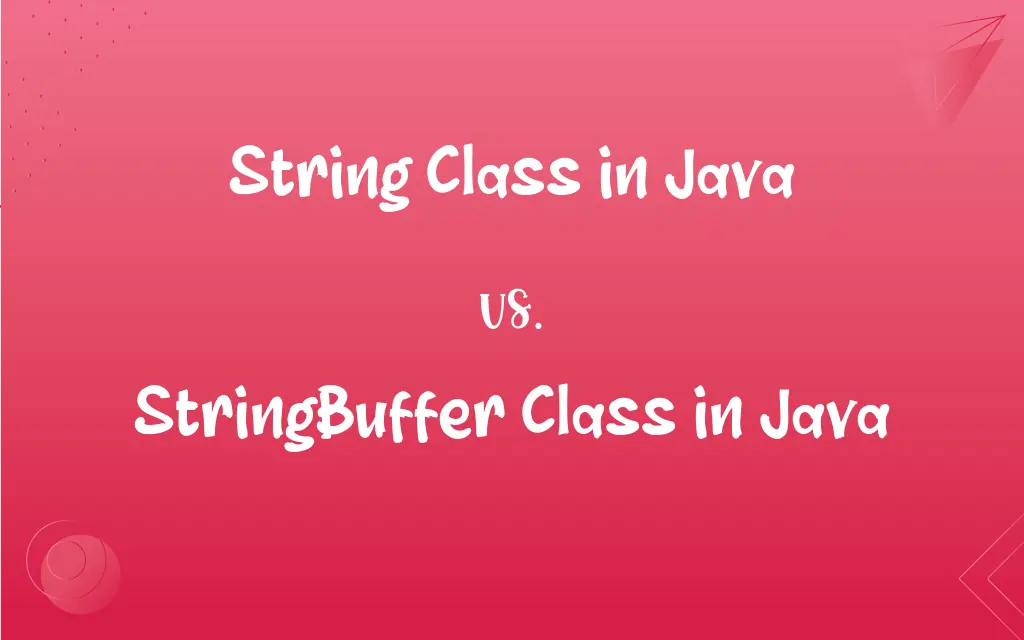
Key Differences
The String class in Java creates immutable string objects, meaning once a String object is created, it cannot be changed. On the other hand, StringBuffer creates mutable string objects, allowing modifications without creating new objects.
Due to immutability, String class operations often require more memory as each modification creates a new String. In contrast, StringBuffer allows in-place modifications, which can be more memory-efficient for frequent changes.
StringBuffer class in Java is thread-safe, meaning it is designed to be safely used in a multi-threaded environment. String class objects are inherently thread-safe due to their immutability but do not have synchronized methods like StringBuffer.
String class is suitable for scenarios where text is not expected to change, like constants. StringBuffer is preferred in scenarios where a string is modified multiple times, such as in loops or when constructing complex strings.
Both String and StringBuffer provide a range of methods for string manipulation. However, StringBuffer has additional methods for appending, inserting, and modifying characters in the existing string buffer.
ADVERTISEMENT
Comparison Chart
Mutability
Immutable - cannot be modified after creation
Mutable - can be modified after creation
Performance
Less efficient for frequently modified strings
More efficient for frequently modified strings
Thread Safety
Thread-safe due to immutability
Thread-safe with synchronized methods
Use Case
Best for constant and unchanging text
Ideal for strings that need frequent modifications
String Modification
Requires creation of a new string for changes
Allows in-place modifications
ADVERTISEMENT
String Class in Java and StringBuffer Class in Java Definitions
String Class in Java
The String class in Java represents immutable sequences of characters.
String greeting = 'Hello World'; defines a string in Java.
StringBuffer Class in Java
StringBuffer is efficient for strings that change frequently.
Using StringBuffer in loops for concatenation improves performance.
String Class in Java
String literals in Java are instances of the String class.
String name = 'Alice'; creates a String object from a literal.
StringBuffer Class in Java
StringBuffer has methods like append, insert, and delete for string manipulation.
Sb.delete(0, 5); modifies the content of the StringBuffer.
String Class in Java
Java's String class is commonly used for text processing.
Parsing input data often involves the String class in Java.
StringBuffer Class in Java
StringBuffer in Java is used for creating and manipulating dynamic strings.
StringBuffer sb = new StringBuffer('Hello'); allows append operations.
String Class in Java
The String class in Java provides methods for string manipulation.
The length() method of String class returns the string's length.
StringBuffer Class in Java
StringBuffer class allows strings to be modified after creation.
Sb.append(' World'); modifies the StringBuffer in place.
String Class in Java
String objects in Java, once created, cannot be altered.
Concatenating strings in Java creates new String objects.
StringBuffer Class in Java
Java's StringBuffer provides thread-safe string operations.
StringBuffer can be safely used in multithreaded applications.
FAQs
What is the primary difference between String and StringBuffer in Java?
String is immutable, while StringBuffer is mutable.
When should I use String class in Java?
Use String when you have fixed or rarely changing text.
How does the memory allocation differ for String and StringBuffer?
String may require more memory for modifications, while StringBuffer uses existing memory more effectively.
Can we replace characters in a String in Java?
You can replace characters, but it creates a new String object.
Can we convert a StringBuffer to a String in Java?
Yes, using the toString() method of StringBuffer.
Is the String class in Java efficient for string concatenation in loops?
No, it's less efficient due to creating many interim String objects.
Is StringBuffer thread-safe in Java?
Yes, StringBuffer is thread-safe with synchronized methods.
How does StringBuffer improve performance in Java?
By allowing in-place modifications, it reduces the need for object creation.
Can StringBuffer in Java change its size?
Yes, StringBuffer can expand or contract as needed.
What are some common methods of StringBuffer in Java?
Common methods include append(), insert(), delete(), and reverse().
What is String interning in Java?
String interning is a method of storing only one copy of each distinct String value.
How do String and StringBuffer handle null in Java?
String methods can throw NullPointerException, while StringBuffer handles null as "null" string.
Can we create a substring in StringBuffer in Java?
Yes, using methods like substring(), which returns a String.
Are String objects in Java stored in the heap or the pool?
String literals are stored in the string pool, while String objects are in the heap.
Are String operations faster than StringBuffer in Java?
For single, unchanging strings, String is faster; for modifications, StringBuffer is better.
Does immutability make String in Java more secure?
Yes, immutability in String prevents unauthorized changes, enhancing security.
How does String handling in Java impact garbage collection?
Frequent String modifications can lead to more garbage collection cycles.
How is the capacity of StringBuffer managed in Java?
StringBuffer automatically increases its capacity when needed.
Do String and StringBuffer have different methods for length?
No, both have a length() method, but they work on different string representations.
Can we directly compare String and StringBuffer objects in Java?
Not directly; StringBuffer needs to be converted to String for comparison.
About Author
Written by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.

Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
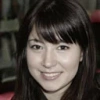