Inline in C++ vs. Macro in C++: What's the Difference?

Edited by Janet White || By Harlon Moss || Published on February 13, 2024
In C++, 'inline' is a keyword for suggesting function inlining to the compiler for efficiency, while 'macro' is a preprocessor directive for text replacement before compilation.

Key Differences
In C++, 'inline' is a keyword used with functions, suggesting the compiler to replace the function call with its code at compile time, potentially reducing call overhead. 'Macro', on the other hand, is defined using the '#define' preprocessor directive, replacing text in the code with defined content before the compilation process.
Inline functions in C++ are handled by the compiler, which decides whether to inline the function based on complexity and size. Macros are processed by the preprocessor, with no understanding of C++ syntax or scope, leading to potential issues with complex expressions.
Inline functions are type-safe and support overloading, ensuring that the correct function is called based on the argument types. Macros are not type-safe and cannot be overloaded, leading to potential type-related issues.
Debugging inline functions in C++ is similar to regular functions, with clear error messages and line numbers. Macros can be challenging to debug due to text replacement, often leading to less clear error messages and line numbers not aligning with original code.
Inline functions are typically used for small, frequently called functions to optimize performance. Macros are used for code that needs to be reused or for conditional compilation, but require caution due to their potential complexity and debugging challenges.
ADVERTISEMENT
Comparison Chart
Purpose
Suggest function inlining for efficiency
Text replacement before compilation
Type Safety
Type-safe
Not type-safe
Overloading
Supports overloading
Cannot be overloaded
Debugging
Easier to debug
More challenging to debug
Compiler Handling
Handled by compiler
Processed by preprocessor
ADVERTISEMENT
Inline in C++ and Macro in C++ Definitions
Inline in C++
'inline' helps reduce function call overhead for small functions.
Inline void logMessage() { cout << Logging; } avoids the overhead of a function call.
Macro in C++
'macro' is useful for conditional compilation and code reuse.
#ifdef DEBUG log(Debugging); #endif uses macro for conditional logging.
Inline in C++
'inline' suggests the compiler to replace a function call with its code.
Inline int add(int a, int b) { return a + b; } allows direct substitution of add function.
Macro in C++
'macro' can make debugging harder due to text replacement.
#define ASSERT(x) if(!(x)) abort(); can be challenging to debug.
Inline in C++
'inline' is part of the function signature and hints at optimization.
Inline double computeArea(double radius) { return 3.14 * radius * radius; } hints at optimizing the area computation.
Macro in C++
'macro' is a preprocessor directive for textual code replacement.
#define PI 3.14 replaces occurrences of PI with 3.14 in the code.
Inline in C++
'inline' functions are subject to the compiler's discretion for actual inlining.
Inline int max(int a, int b) { return (a > b) ? a : b; } may or may not be inlined based on the compiler's decision.
Macro in C++
'macro' can lead to unexpected results due to lack of type safety.
#define SQUARE(x) x*x can yield incorrect results for SQUARE(1 + 2).
Inline in C++
'inline' functions retain type safety and can be overloaded.
Inline float add(float a, float b) { return a + b; } overloads the add function for float types.
Macro in C++
'macro' does not understand C++ scope or syntax.
#define BEGIN { may cause issues due to scope misunderstanding.
FAQs
Can inline functions be used for optimization?
Yes, inline functions can optimize performance by reducing function call overhead.
What is a macro in C++?
A macro in C++ is a preprocessor directive for replacing text in the code.
When should you use inline functions over macros?
Use inline functions for type safety and easier debugging, especially with small, frequently called functions.
Is it possible to debug macro definitions?
Debugging macro definitions can be challenging due to their text replacement nature.
What are the disadvantages of using macros?
Macros can lead to issues like lack of type safety, debugging difficulties, and unexpected behaviors due to text replacement.
Can macros be used for conditional compilation?
Yes, macros are often used for conditional compilation and code reuse.
Are macros type-safe in C++?
No, macros are not type-safe and can lead to type-related errors.
How does the compiler decide to actually inline a function?
The compiler decides based on factors like function complexity and size.
What is an inline function in C++?
An inline function suggests the compiler to replace the function call with its code.
Do inline functions support function overloading?
Yes, inline functions support overloading like regular functions.
How do macros affect compilation time?
Macros can increase compilation time due to the preprocessor's text replacement process.
Can macros affect the readability of the code?
Yes, extensive use of macros can make the code less readable and harder to maintain.
Are inline functions always inlined by the compiler?
No, it's up to the compiler's discretion to inline a function or not.
What happens if a compiler ignores the inline request?
If a compiler ignores the inline request, the function is treated as a normal function call.
Can macros be used to define constants?
Yes, macros are often used to define constants like #define PI 3.14159.
Can macros introduce errors during preprocessor expansion?
Yes, macros can introduce errors or unexpected behavior due to their text replacement mechanism.
Are inline functions part of C++ standard?
Yes, inline functions are a standard feature in C++.
Are inline functions more efficient than regular functions?
Inline functions can be more efficient for small functions by eliminating call overhead.
Do macros participate in C++ name mangling?
No, macros are replaced before the compilation stage and do not participate in name mangling.
How do inline functions affect memory usage?
Inline functions may increase memory usage if the compiler decides to inline them extensively.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
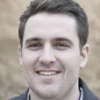
Edited by
Janet WhiteJanet White has been an esteemed writer and blogger for Difference Wiki. Holding a Master's degree in Science and Medical Journalism from the prestigious Boston University, she has consistently demonstrated her expertise and passion for her field. When she's not immersed in her work, Janet relishes her time exercising, delving into a good book, and cherishing moments with friends and family.
