Final in Java vs. Finally in Java: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 16, 2024
In Java, final is a keyword for declaring constants or restricting inheritance, while finally is a block that executes code irrespective of exception handling.

Key Differences
The final keyword in Java is used to declare a variable as a constant, meaning its value cannot be modified once initialized. It can also be used with classes and methods to prevent them from being subclassed or overridden, respectively. Finally, on the other hand, is a block used in exception handling that executes code regardless of whether an exception is thrown or caught.
A final variable must be initialized when declared or in the constructor if it’s an instance variable. This ensures the immutability of the variable. The finally block is typically used to close resources like files or database connections, ensuring these actions occur even if an error happens in the try-catch blocks.
When a class is declared as final, it cannot be extended by any subclass, ensuring the class’s behavior cannot be altered. Methods declared as final cannot be overridden by subclasses. The finally block does not affect inheritance or method behavior but guarantees the execution of important cleanup code.
A final method ensures reliability and consistency, particularly for methods with critical functionality that should not be changed by subclasses. The finally block is a key feature for robust exception handling, ensuring resources are properly released, and cleanup operations are performed, maintaining system stability.
The use of final can optimize performance, as the compiler knows these elements are immutable. The use of finally ensures better resource management and program reliability, especially in the face of unexpected errors or exceptions.
ADVERTISEMENT
Comparison Chart
Purpose
Declaring constants, restricting inheritance
Executing code irrespective of exception handling
Usage
With variables, methods, and classes
In try-catch blocks for exceptions
Effect
Prevents modification or extension
Ensures execution of code for cleanup and resource management
Initialization
Variables must be initialized
No initialization needed; part of exception handling
Application
Ensuring immutability and consistency in code
Guaranteeing resource release and cleanup actions
ADVERTISEMENT
Final in Java and Finally in Java Definitions
Final in Java
Disallows class inheritance.
Public final class ImmutableClass { ... }
Finally in Java
Executes code after a try-catch block.
Try { ... } catch (Exception e) { ... } finally { ... }
Final in Java
Used to declare a constant variable.
Final int MAX_CONNECTIONS = 5;
Finally in Java
Runs irrespective of exception occurrence.
Finally { System.out.println(Cleanup done); }
Final in Java
Prevents method overriding.
Public final void compute() { ... }
Finally in Java
Provides robustness in exception handling.
Finally { threadPool.shutdown(); }
Final in Java
Ensures variable immutability.
Final String USERNAME = user123;
Finally in Java
Commonly used for releasing resources.
Finally { databaseConnection.disconnect(); }
Final in Java
Enhances performance due to immutability.
Final double PI = 3.14159;
Finally in Java
Ensures execution of cleanup code.
Finally { file.close(); }
FAQs
What does final indicate in Java?
It marks a variable, method, or class as immutable or unchangeable.
Does finally always execute in Java?
Yes, except in cases of JVM crash or System.exit() call.
Can a final class be extended?
No, a final class cannot be subclassed.
What happens if an exception is thrown in a finally block?
The exception disrupts the normal flow, potentially overriding previous exceptions.
Can a final variable be reassigned?
No, once a final variable is assigned, it cannot be changed.
How does final improve performance in Java?
The compiler can optimize final variables, knowing they won’t change.
When should you use finally in Java?
When you need to execute cleanup code, like closing resources.
What is the purpose of a finally block?
To execute code after a try-catch block, regardless of an exception's occurrence.
What is a final parameter?
A method parameter declared as final cannot be modified within the method.
Is finally block executed on try without exception?
Yes, it executes regardless of an exception.
Can final and abstract be used together?
No, as final prevents method overriding, which is the purpose of abstract.
Is a finally block required with try-catch?
No, it's optional but recommended for proper resource management.
Is it mandatory to initialize a final variable at declaration?
Yes, unless it's an instance variable, which can be initialized in the constructor.
Can finally be used without a catch block?
Yes, it can follow a try block directly.
What is the benefit of declaring a method final?
It prevents the method from being overridden in subclasses.
What is the difference between finally and finalize in Java?
finally is for code execution, while finalize is a method called by the garbage collector.
Can finally block contain a return statement?
Yes, but it's not recommended as it can lead to confusing code.
Can a final variable be static?
Yes, final variables can be static, and they are constants at the class level.
Can we catch exceptions in a finally block?
No, finally is not for catching exceptions but for ensuring code execution.
What happens if a final variable is not initialized?
The code will not compile, as final variables must be initialized.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
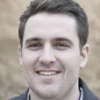
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
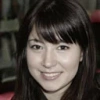