Static in Java vs. Final in Java: What's the Difference?

Edited by Aimie Carlson || By Harlon Moss || Published on February 14, 2024
In Java, static indicates a class-level member shared by all instances, while final denotes a constant that cannot be changed once initialized.

Key Differences
In Java, static is a keyword used to define class-level properties and methods that belong to the class rather than any individual instance. In contrast, final is used to declare constants or methods that cannot be overridden, or classes that cannot be subclassed.
A static variable is shared among all instances of a class, meaning it holds the same value across all instances. On the other hand, a final variable, once assigned a value, cannot have its value changed.
static methods can be called without creating an instance of the class, making them useful for utility or helper methods. Conversely, a final method cannot be overridden by any subclass, ensuring consistent behavior.
A static block in Java is used for static initialization of a class. In contrast, a final class cannot be extended, preserving the class's integrity and preventing inheritance.
static members belong to the class rather than a specific instance, which is efficient for memory management. final is used to enforce immutability, enhancing security and design clarity.
ADVERTISEMENT
Comparison Chart
Purpose
Defines class-level members shared by all instances
Declares constants, non-overridable methods, or non-extendable classes
Usage in Variables
Shared value among all instances
Value cannot be changed after initialization
Usage in Methods
Can be called without an instance
Cannot be overridden by subclasses
Memory Management
Efficient for variables used by all instances
Ensures immutability and security
Usage in Classes
Cannot be used to define classes
Prevents a class from being subclassed
ADVERTISEMENT
Static in Java and Final in Java Definitions
Static in Java
Static keyword in Java is used to indicate that a member variable or method belongs to a class, rather than instances of the class.
Public static int count; - count is a static variable shared by all instances of the class.
Final in Java
Final classes cannot be subclassed.
Public final class ImmutableClass {} - ImmutableClass cannot be extended.
Static in Java
Static variables are stored in static memory, which is common to all instances.
Public static String name = Java; - name is a static variable shared by all objects.
Final in Java
Final keyword in Java is used to declare a constant variable that cannot be modified after it is initialized.
Final int MAX_SIZE = 100; - MAX_SIZE is a constant that cannot be changed.
Static in Java
Static can be used to define a static block for initializing class variables.
Static { System.loadLibrary(lib); } - This static block loads a library once for the class.
Final in Java
A final variable must be initialized before the constructor completes.
Final double PI; - PI must be initialized in the constructor.
Static in Java
Static members are initialized only once at the start of the execution.
Static { count = 0; } - This static block sets the initial value of count.
Final in Java
Final can be used to prevent method overriding in subclasses.
Public final void display() {} - display method cannot be overridden.
Static in Java
Static methods can be accessed without creating an object of the class.
Public static void printCount() { System.out.println(count); } - printCount can be called without an object.
Final in Java
Final parameters in a method cannot be modified within the method.
Void calculate(final double interestRate) {} - interestRate parameter cannot be changed in the method.
FAQs
Can a static method access instance variables?
No, static methods cannot directly access instance variables.
What is a static variable in Java?
A static variable is a class-level variable shared by all instances of the class.
Are static members part of objects in Java?
No, static members belong to the class and are not part of individual objects.
Can a final method be overridden?
No, a final method cannot be overridden in any subclass.
Can final variables be reassigned?
No, once assigned, final variables cannot be reassigned.
Can static methods be overridden?
Static methods cannot be overridden but can be hidden by a subclass.
Why use static variables in Java?
Static variables are used for values shared across all instances, saving memory.
How is memory managed for static variables?
Static variables are stored in static memory, common to all class instances.
Can a final variable be left uninitialized?
A final variable must be initialized at the time of declaration or in the constructor.
What is a final variable in Java?
A final variable is a constant that cannot be modified once it is initialized.
Can constructors be final in Java?
No, constructors cannot be declared as final in Java.
What happens when a static method is inherited?
When inherited, the static method belongs to the superclass and is not part of the subclass.
What is the purpose of final classes in Java?
Final classes are used to prevent them from being subclassed.
How are static variables initialized?
Static variables are initialized at class loading time, often through a static block.
Does final ensure immutability?
Final ensures a variable cannot be reassigned, but doesn't guarantee immutability of the object it references.
Is it possible to declare a class static in Java?
Only nested classes can be declared static, not top-level classes.
Can final and static be used together?
Yes, a variable can be declared as both final and static.
Why use final parameters in methods?
Final parameters ensure that the value passed to a method remains constant.
About Author
Written by
Harlon MossHarlon is a seasoned quality moderator and accomplished content writer for Difference Wiki. An alumnus of the prestigious University of California, he earned his degree in Computer Science. Leveraging his academic background, Harlon brings a meticulous and informed perspective to his work, ensuring content accuracy and excellence.
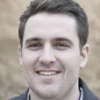
Edited by
Aimie CarlsonAimie Carlson, holding a master's degree in English literature, is a fervent English language enthusiast. She lends her writing talents to Difference Wiki, a prominent website that specializes in comparisons, offering readers insightful analyses that both captivate and inform.
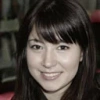